In this post, we will learn how to multiply two matrices using multi-dimensional arrays in C Programming language.
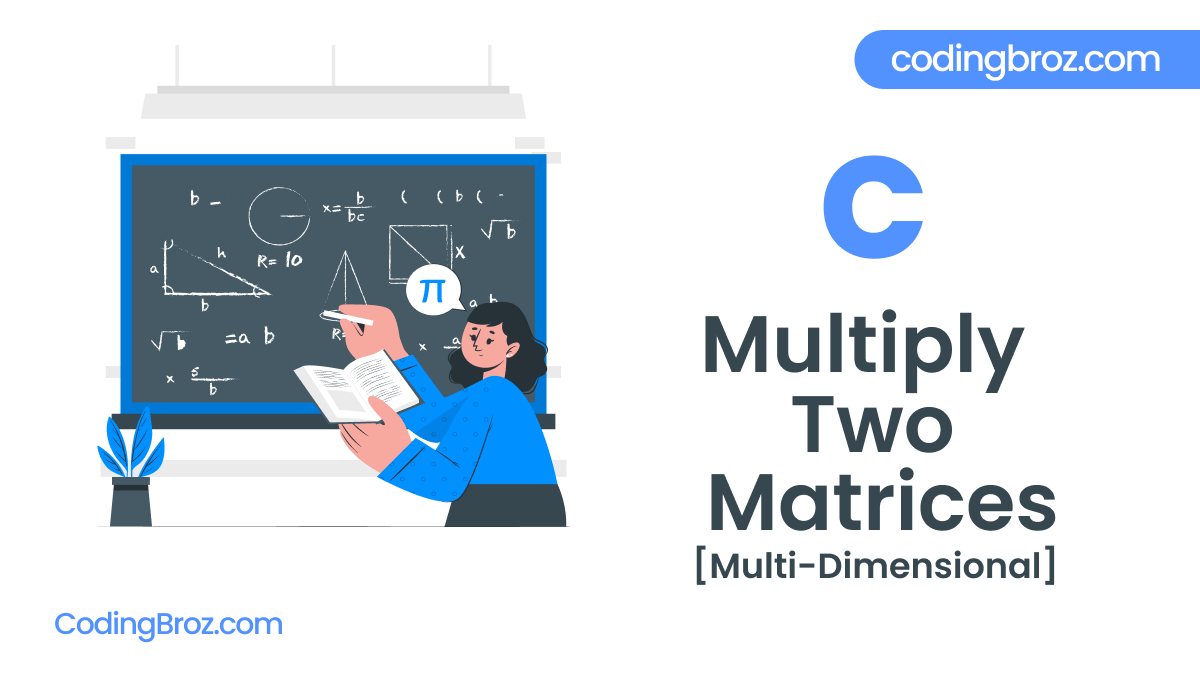
To multiply two matrices, we have to follow certain rules. Those rules are as follows:-
- Make sure that the no. of column in the first matrix equals the no. of rows in the second matrix
- Multiply the elements of each row of the first matrix by the elements of each column in the second matrix
- Add the Products
- Place the added products in the respective column
We will be using the above rules in our program to compute the multiplication of two matrices.
So, without further ado, let’s begin this tutorial.
C Program To Multiply Two Matrices Using Multi-dimensional Arrays
#include <stdio.h> int main(){ int i, j, k, sum = 0, row1, column1, row2, column2; int first[10][10], second[10][10], result[10][10]; //Asking for input printf("Enter no. of rows and columns of first matrix: /n"); scanf("%d %d", &row1, &column1); printf("Enter no. of rows and columns of second matrix: /n"); scanf("%d %d", &row2, &column2); if (column1 != row2) printf("Error!! Multiplication is not possible."); else{ printf("Enter elements of first matrix: \n"); for(i = 0; i < row1; i++) for(j = 0; j < column1; j++) scanf("%d", &first[i][j]); printf("Enter elements of second matrix: \n"); for (i = 0; i < row2; i++) for (j = 0; j < column2; j++) scanf("%d", &second[i][j]); //Calculating the multiplication for (i = 0; i < row1; i++) { for (j = 0; j < column2; j++) { for (k = 0; k < row2; k++) { sum = sum + first[i][k] * second[k][j]; } result[i][j] = sum; sum = 0; } } //Displaying the result printf("Multiplication of two matrices are: \n"); for (i = 0; i < row1; i++) { for (j = 0; j < column2; j++) printf("%d \t", result[i][j]); printf("\n"); } } return 0; }
Output
Enter no. of rows and columns of first matrix: 2 2
Enter no. of rows and columns of second matrix: 2 2
Enter elements of first matrix:
1 2
3 4
Enter elements of second matrix:
2 1
4 3
Multiplication of two matrices are:
10 7
22 15
How Does This Program Work ?
int i, j, k, sum = 0, row1, column1, row2, column2; int first[10][10], second[10][10], result[10][10];
In this program, we have declared some int data type variables which will store the elements of the two matrices.
//Asking for input printf("Enter no. of rows and columns of first matrix: /n"); scanf("%d %d", &row1, &column1); printf("Enter no. of rows and columns of second matrix: /n"); scanf("%d %d", &row2, &column2);
Then, the user is asked to enter the no. of rows and columns of the two matrices.
if (column1 != row2) printf("Error!! Multiplication is not possible.");
If no. of columns of the first matrix = no. of rows of the second matrix, then Multiplication of the two matrices is possible. Otherwise, our program will display the message that “Multiplication is not possible“.
printf("Enter elements of first matrix: \n"); for(i = 0; i < row1; i++) for(j = 0; j < column1; j++) scanf("%d", &first[i][j]); printf("Enter elements of second matrix: \n"); for (i = 0; i < row2; i++) for (j = 0; j < column2; j++) scanf("%d", &second[i][j]);
Now, the user is asked to enter the elements of the first and second matrix.
//Calculating the multiplication for (i = 0; i < row1; i++) { for (j = 0; j < column2; j++) { for (k = 0; k < row2; k++) { sum = sum + first[i][k] * second[k][j]; } result[i][j] = sum; sum = 0; } }
We calculate the multiplication of the two matrices using a simple for loop.
//Displaying the result printf("Multiplication of two matrices are: \n"); for (i = 0; i < row1; i++) { for (j = 0; j < column2; j++) printf("%d \t", result[i][j]); printf("\n"); } }
Finally, the end product is displayed to the screen using printf() function.
Conclusion
I hope after going through this post, you understand how to multiply two matrices using multi-dimensional arrays in C Programming language.
If you have any doubt regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: