In this post, we will learn how to add two fractions in C Programming language.
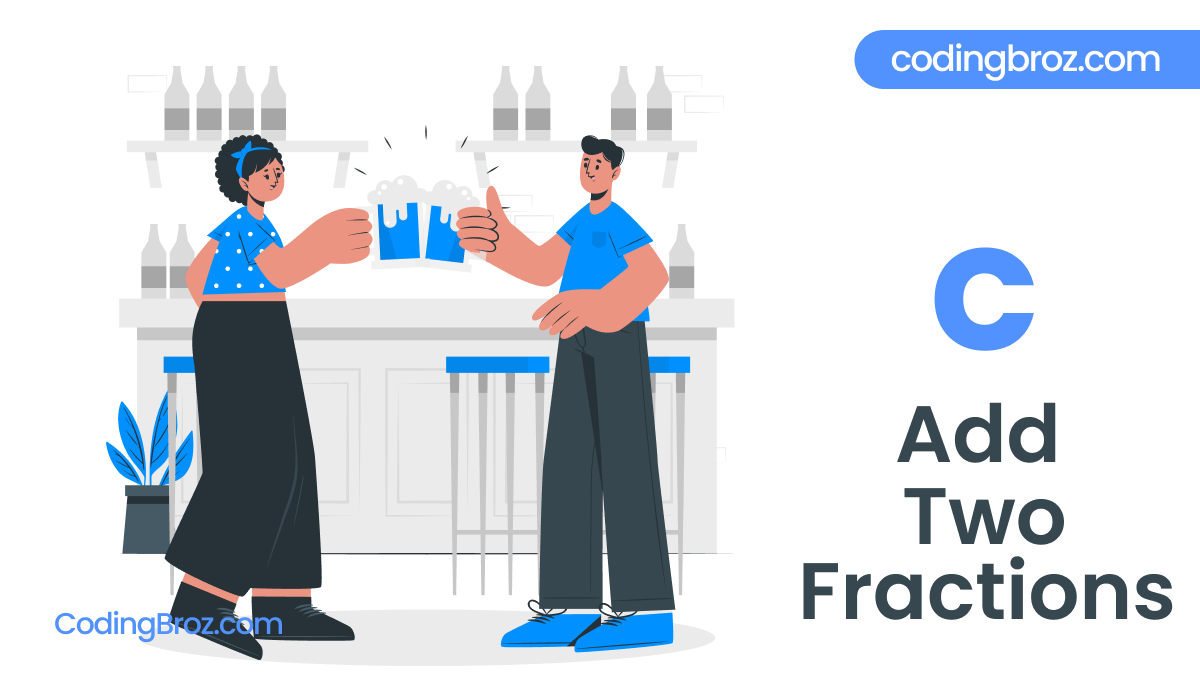
A Fraction is a number that represents part of a whole. A fraction is a number of the form a / b, where a and b are integers and b != 0.
To Add Fraction there are three simple steps:
- Make sure the denominators are the same.
- Add the numerators.
- Simply the fraction if possible.
This program takes the fraction as input from the user and then adds the fractions to get the desired result.
So, without further ado, let’s begin this tutorial.
C Program To Add Two Fractions
// C Program To Add Two Fractions #include <stdio.h> int main(){ int a, b, c, d, x, y, gcd; // Asking for input printf("Enter the numerator[First Number]: "); scanf("%d", &a); printf("Enter the denominator[First Number]: "); scanf("%d", &b); printf("Enter the numerator[Second Number]: "); scanf("%d", &c); printf("Enter the denominator[Second Number]: "); scanf("%d", &d); x = (a * d + b * c); y = b * d; for (int i = 1; i <= x && i <= y; ++i){ if (x % i == 0 && y % i == 0){ gcd = i; } } printf("After addition we get: %d/%d +%d/%d = %d/%d", a, b, c, d, x/gcd, y/gcd); return 0; }
Output
Enter the numerator[First Number]: 3
Enter the denominator[First Number]: 4
Enter the numerator[Second Number]: 4
Enter the denominator[Second Number]: 5
After addition we get: 3/4 +4/5 = 31/20
How Does This Program Work ?
int a, b, c, d, x, y, gcd;
In this program, we have declared 7 int data type variables named as a, b, c, d, x, y and gcd respectively.
// Asking for input
printf("Enter the numerator[First Number]: ");
scanf("%d", &a);
printf("Enter the denominator[First Number]: ");
scanf("%d", &b);
printf("Enter the numerator[Second Number]: ");
scanf("%d", &c);
printf("Enter the denominator[Second Number]: ");
scanf("%d", &d);
Then, the user is asked to enter the values of fraction.
x = (a * d + b * c);
y = b * d;
We multiply the numerator of the first fraction to the denominator of the second fraction and the numerator of the second fraction to the denominator of the first fraction.
We add the results and store them in the x named variable.
Then, denominators are multiplied by each other and stored in y named variable.
for (int i = 1; i <= x && i <= y; ++i){
if (x % i == 0 && y % i == 0){
gcd = i;
}
}
Then, we calculate the GCD of x and y (if any).
printf("After addition we get: %d/%d +%d/%d = %d/%d", a, b, c, d, x/gcd, y/gcd);
Finally, we divide the fraction x/y by the gcd obtained and print the result using printf() function.
Conclusion
I hope after going through this post, you understand how to add two fractions using C Programming language.
If you have any doubt regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: