In this post, we will learn how to add two matrices using multi-dimensional arrays using C Programming language.
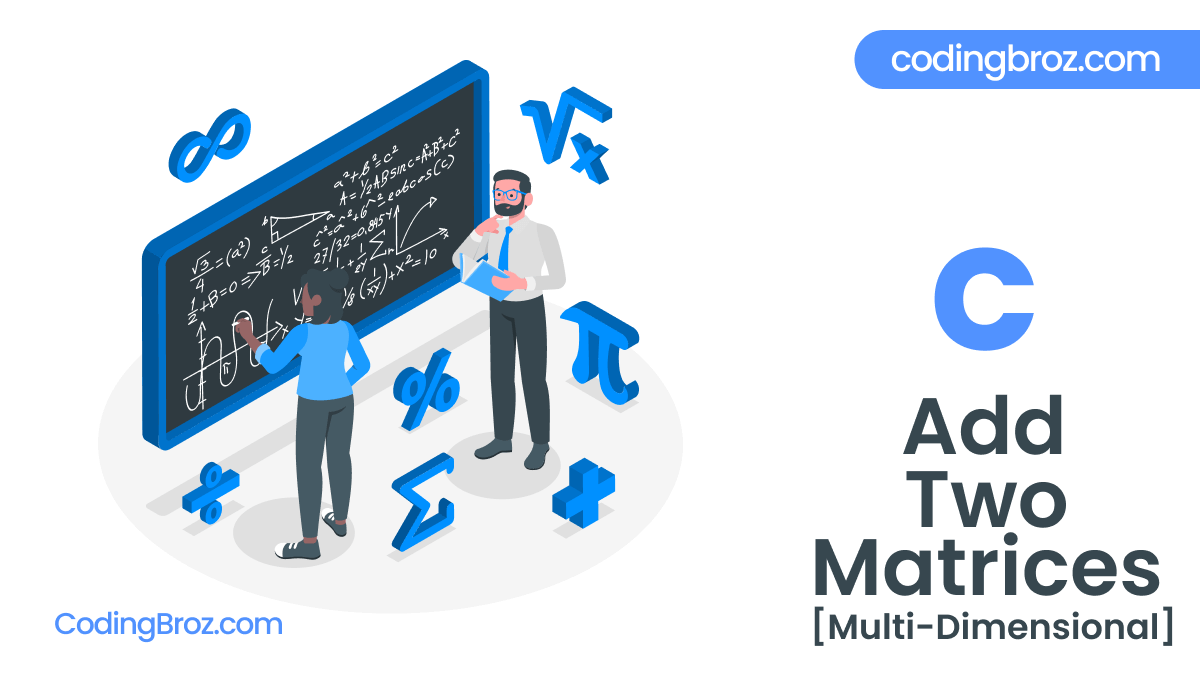
A Matrix is a rectangular array of numbers that is arranged in the form of rows and columns. For example: A Matrix of order 3 x 3 look like this:
1 3 5
4 5 7
5 9 2
To Add Matrices, there are certain rules:
- Matrices should be of same dimension (e.g. – Same no. of rows and columns)
- Matrix Addition is commutative (i.e. A + B = B + A)
- Matrix Addition is Associative (i.e. (A + B) + C = A + (B + C))
We will keep these rules in mind while adding the matrices.
So, without further ado, let’s begin this tutorial.
C Program To Add Two Matrices Using Multi-dimensional Arrays
// C Program To Add Two Matrices Using Multi-dimensional Arrays #include <stdio.h> int main(){ int a[10][10], b[10][10], sum[10][10], i, j,column, row; // Asking for input printf("Enter total no. of rows[Between 1 and 10]: "); scanf("%d", &row); printf("Enter total no. of columns[Between 1 and 10]: "); scanf("%d", &column); printf("Enter First Matrix: \n"); for (i = 0; i < row; i++) for(j = 0; j < column; j++) scanf("%d", &a[i][j]); printf("Enter Second Matrix: \n"); for (i = 0; i < row; i++) for (j = 0; j < column; j++) scanf("%d", &b[i][j]); // Adding Two Matrices for (i = 0; i < row; i++){ for (j = 0; j < column; j++){ sum[i][j] = a[i][j] + b[i][j]; } } // Printing the result printf("Sum of Two Matrices: \n"); for (i = 0; i < row; i++){ for(j = 0; j < column; j++){ printf("%d ", sum[i][j]); } printf("\n"); } return 0; }
Output
Enter total no. of rows[Between 1 and 10]: 3
Enter total no. of columns[Between 1 and 10]: 3
Enter First Matrix:
1 3 5
1 4 6
2 3 5
Enter Second Matrix:
7 4 1
2 4 7
5 2 1
Sum of Two Matrices:
8 7 6
3 8 13
7 5 6
How Does This Program Work ?
int a[10][10], b[10][10], sum[10][10], i, j,column, row;
In this program, we have declared some variables which will store the user inputs.
// Asking for input printf("Enter total no. of rows[Between 1 and 10]: "); scanf("%d", &row); printf("Enter total no. of columns[Between 1 and 10]: "); scanf("%d", &column); printf("Enter First Matrix: \n"); for (i = 0; i < row; i++) for(j = 0; j < column; j++) scanf("%d", &a[i][j]); printf("Enter Second Matrix: \n"); for (i = 0; i < row; i++) for (j = 0; j < column; j++) scanf("%d", &b[i][j]);
Now, the user is asked to enter the total no. of rows and columns. The user is also asked to enter the element of each of the matrices.
// Adding Two Matrices for (i = 0; i < row; i++){ for (j = 0; j < column; j++){ sum[i][j] = a[i][j] + b[i][j]; } }
Now, we add the elements of matrices with each other.
// Printing the result printf("Sum of Two Matrices: \n"); for (i = 0; i < row; i++){ for(j = 0; j < column; j++){ printf("%d ", sum[i][j]); }
Finally, the sum of two matrices is printed using the printf() function.
Conclusion
I hope after going through this post, you understand how to add two matrices using multi-dimensional arrays in C Programming language.
If you have any doubt regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: