In this post, we will learn how to add two binary numbers in C Programming language.
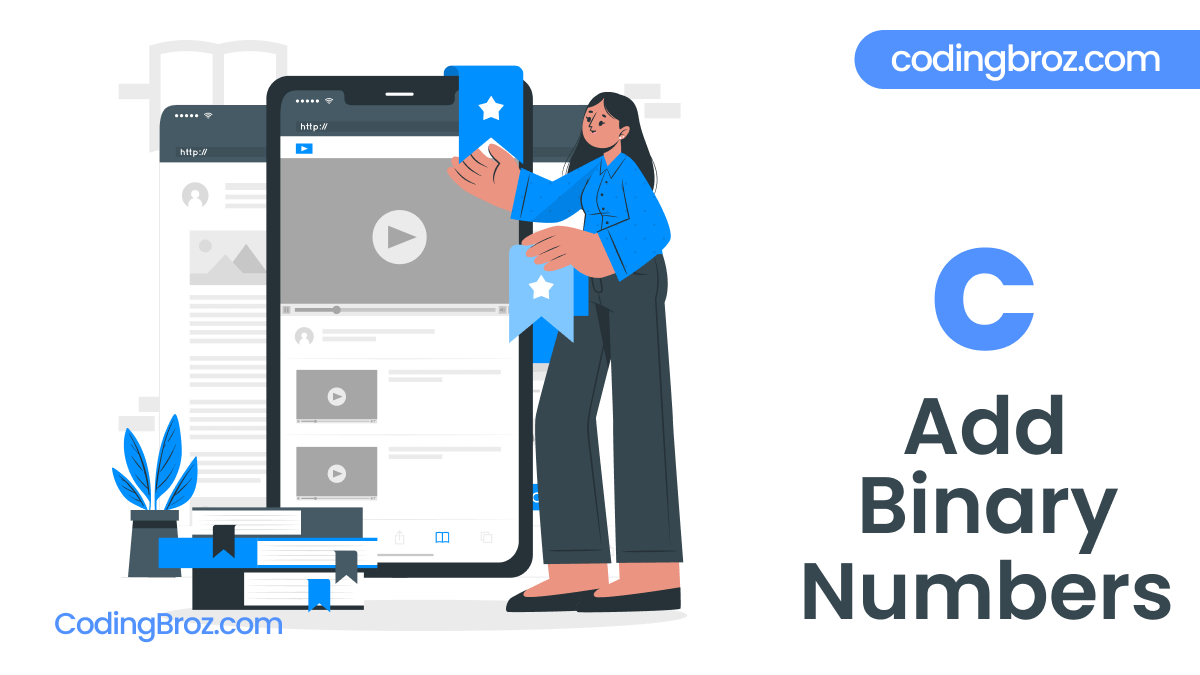
Binary numbers are those numbers which can be expressed in the base-2 numeral system, a mathematical expression which uses only two symbols: ‘0’ and ‘1’.
The binary addition rules are as follows:
- 0 + 0 = 0
- 0 + 1 = 1
- 1 + 0 = 1
- 1 + 1 = 10 which is 0 carry 1
We will be using these rules in our program to calculate the sum of two binary numbers.
So, without further ado, let’s begin this tutorial.
C Program To Add Two Binary Numbers
// C Program To Add Two Binary Numbers #include <stdio.h> int main(){ long int binary1, binary2; int i = 0, rem = 0, sum[20]; // Asking for Input printf("Enter first binary number: "); scanf("%ld", &binary1); printf("Enter second binary number: "); scanf("%ld", &binary2); while (binary1 != 0 || binary2 != 0){ sum[i++] = (binary1 %10 + binary2 % 10 + rem) % 2; rem = (binary1 %10 + binary2 % 10 + rem) / 2; binary1 = binary1 / 10; binary2 = binary2 / 10; } if (rem != 0) sum[i++] = rem; --i; printf("Sum of two binary numbers is: "); while (i >= 0){ printf("%d", sum[i--]); } return 0; }
Output
Enter first binary number: 10101
Enter second binary number: 11100
Sum of two binary numbers is: 110001
How Does This Program Work ?
long int binary1, binary2; int i = 0, rem = 0, sum[20];
In this program, we have declared two long data type variables named binary1 and binary2. We have also initialized the variables i and rem to 0.
// Asking for Input printf("Enter first binary number: "); scanf("%ld", &binary1); printf("Enter second binary number: "); scanf("%ld", &binary2);
Then, the user is asked to enter the values of two binary numbers. These values will be stored in binary1 and binary2 named variables respectively.
while (binary1 != 0 || binary2 != 0){ sum[i++] = (binary1 %10 + binary2 % 10 + rem) % 2; rem = (binary1 %10 + binary2 % 10 + rem) / 2; binary1 = binary1 / 10; binary2 = binary2 / 10; } if (rem != 0){ sum[i++] = rem; } --i; printf("Sum of two binary numbers is: "); while (i >= 0){ printf("%d", sum[i--]); }
Now, we build up a simple logic which will add the two binary numbers. The result will be printed using printf() function.
Conclusion
I hope after going through this post, you understand how to add two binary numbers in C Programming language.
If you have any doubts regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read:
What does (–i) do in the above program ?