In this program, we will learn how to convert Fahrenheit to Celsius using the C Programming language.
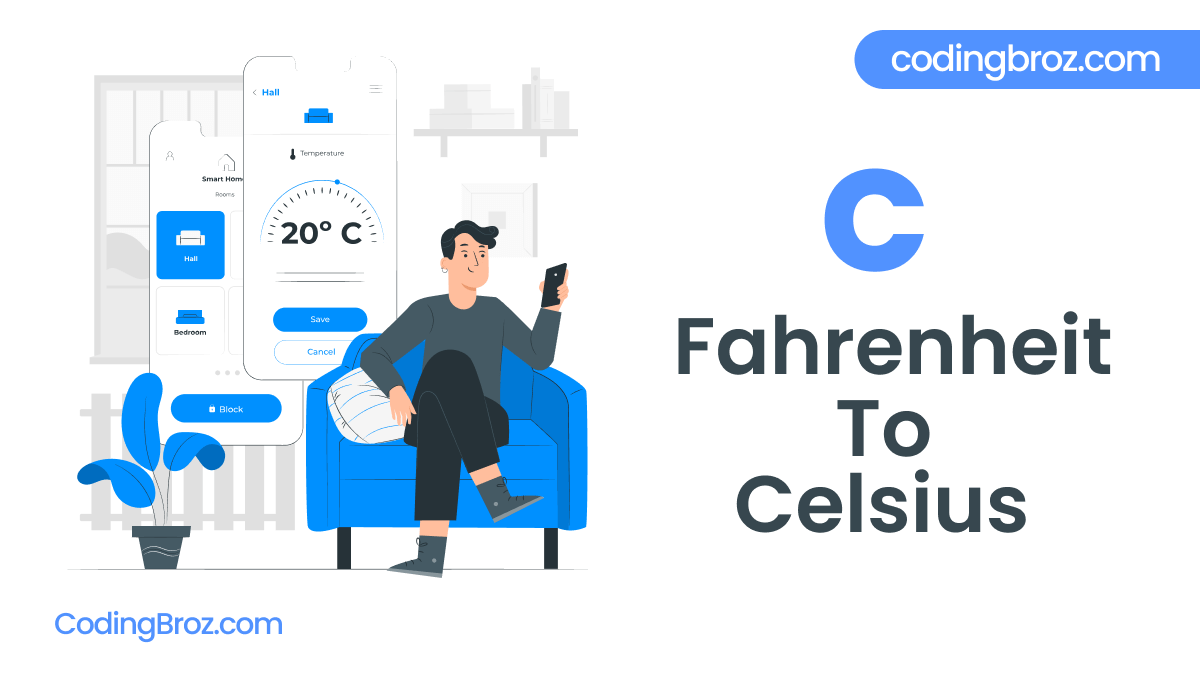
Fahrenheit and Celsius are the scales of temperature. Temperature scales measure how cold or hot a body is.
On the Fahrenheit Temperature scale, water freezes at 32° Fahrenheit and boils at 212° Fahrenheit.
Similarly, on the Celsius scale, water freezes at 0° Celsius and boils at 100° Celsius.
To convert Fahrenheit to celsius, follow these steps:
- Firstly subtract 32 from the Fahrenheit temperature.
- Multiply this particular number by 5
- Finally, divide the number which is achieved by 9
This formula can also be written as °C = (°F – 32) x 5/9.
We will be using the above formula in our program to convert Fahrenheit to Celsius.
So, without further ado, let’s begin this tutorial.
C Program To Convert Fahrenheit To Celsius
//C Program To Convert Fahrenheit To Celsius #include <stdio.h> int main(){ float celsius, fahrenheit; //Asking for input printf("Enter the temperature in Fahrenheit: "); scanf("%f", &fahrenheit); //Fahrenheit to Celsius celsius = (fahrenheit - 32) * 5 / 9; //Displaying the result printf("%.2f in Fahrenheit = %.2f in Celsius", fahrenheit, celsius); return 0; }
Output
Enter the temperature in Fahrenheit: 98.60
98.60 in Fahrenheit = 37.00 in Celsius
How Does This Program Work ?
float celsius, fahrenheit;
In this program, we have declared two float data type variables named celsius and fahrenheit.
//Asking for input printf("Enter the temperature in Fahrenheit: "); scanf("%f", &fahrenheit);
Then, the user is asked to enter the value of temperature in Fahrenheit.
//Fahrenheit to Celsius celsius = (fahrenheit - 32) * 5 / 9;
Now, we convert the temperature into degree Celsius using the formula C = (F – 32) x 5/9.
//Displaying the result printf("%.2f in Fahrenheit = %.2f in Celsius", fahrenheit, celsius);
Finally, the result is printed on the screen using printf() function. Here, we have used %.2f format specifier because we want to display our result only till 2 decimal places.
Conclusion
I hope after going through this post, you understand how to convert fahrenheit to celsius using C Programming language.
If you have any doubt regarding the problem, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: