In this post, we will learn how to find the largest of two numbers using C Programming language.
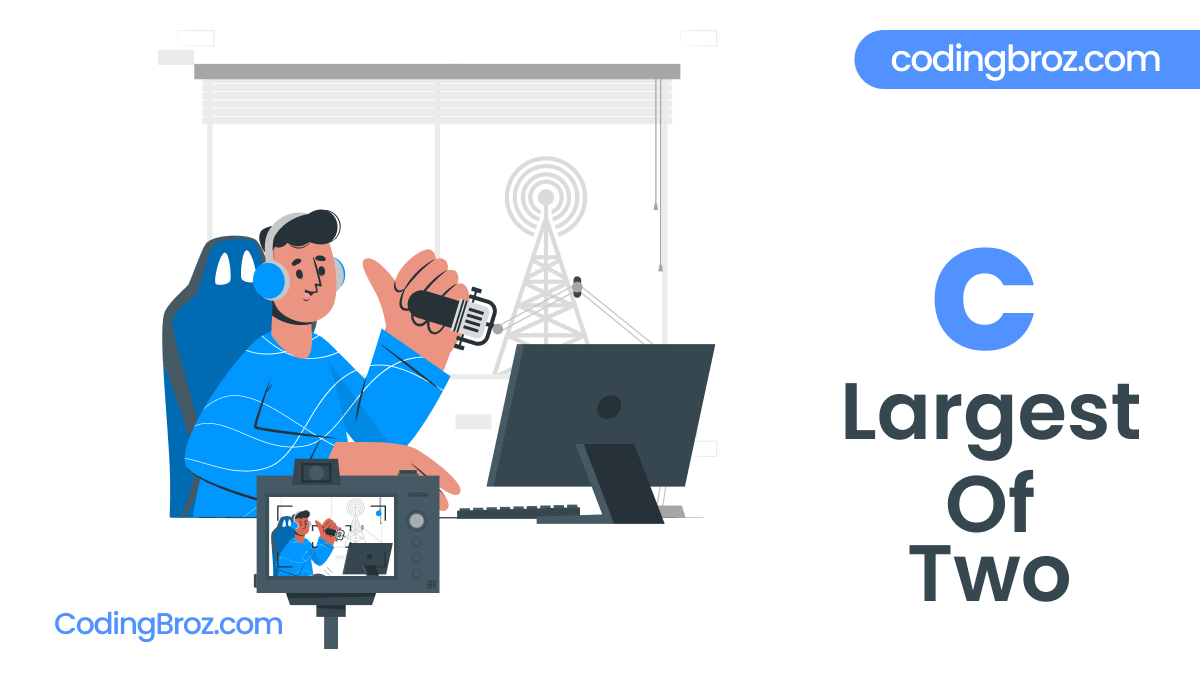
We will be using three different approaches to write the program. The three different methods are as follow:
- Using If-Else Statement
- Using Switch…case Statement
- Using Conditional Operator
So, without further ado, let’s begin the tutorial.
C Program To Find Largest of Two Numbers
// C Program To Find Largest of Two Numbers #include <stdio.h> int main(){ int a, b; // Asking for Input printf("Enter first number: "); scanf("%d", &a); printf("Enter second number: "); scanf("%d", &b); if (a > b){ printf("%d is largest Number.", a); } else if (b > a){ printf("%d is largest Number.", b); } else{ printf("Both numbers are equal."); } return 0; }
Output
Enter first number: 7
Enter second number: 12
12 is the largest Number.
How Does This Program Work ?
int a, b;
In this program, we have declared two int data type variables named as a and b.
// Asking for Input
printf("Enter first number: ");
scanf("%d", &a);
printf("Enter second number: ");
scanf("%d", &b);
Then, the user is asked to enter the value of two numbers.
if (a > b){
printf("%d is Largest Number.", a);
}
else if (b > a){
printf("%d is Largest Number.", b);
}
else{
printf("Both numbers are equal.");
}
Then, we check whether the first number is greater than the second number or not, if yes then the first number is the largest number.
If the first condition is false and the second number is greater than the first one. Then, the second number is the largest number and we’ll print it.
And, if neither of the two conditions satisfy, then it means both numbers are equal.
C Program To Find Largest of Two Numbers Using switch…case
// C Program To Find Largest of Two Numbers Using switch...case #include <stdio.h> int main(){ int a, b; // Asking for Input printf("Enter first number: "); scanf("%d", &a); printf("Enter second number: "); scanf("%d", &b); switch (a > b){ case 0: printf("%d is largest", b); break; case 1: printf("%d is largest", a); break; } return 0; }
Output
Enter first number: 11
Enter second number: 18
18 is largest
C Program To Find Largest of Two Numbers Using Conditional Operator
// C Program To Find Largest of Two Numbers Using Conditional Operator #include <stdio.h> int main(){ int a, b, greatest; // Asking for Input printf("Enter first number: "); scanf("%d", &a); printf("Enter second number: "); scanf("%d", &b); if (a == b){ printf("Both numbers are equal."); } else { greatest = (a > b) ? a : b; printf("%d is the largest number.", greatest); } return 0; }
Output
Enter first number: 15
Enter second number: 8
15 is the largest number.
Conclusion
I hope after going through this post, you understand how to find the largest of two numbers using C Programming language.
If you have any doubt regarding the problem, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: