In this post, we will learn how to check whether a number is divisible by 5 and 11 using C Programming language.
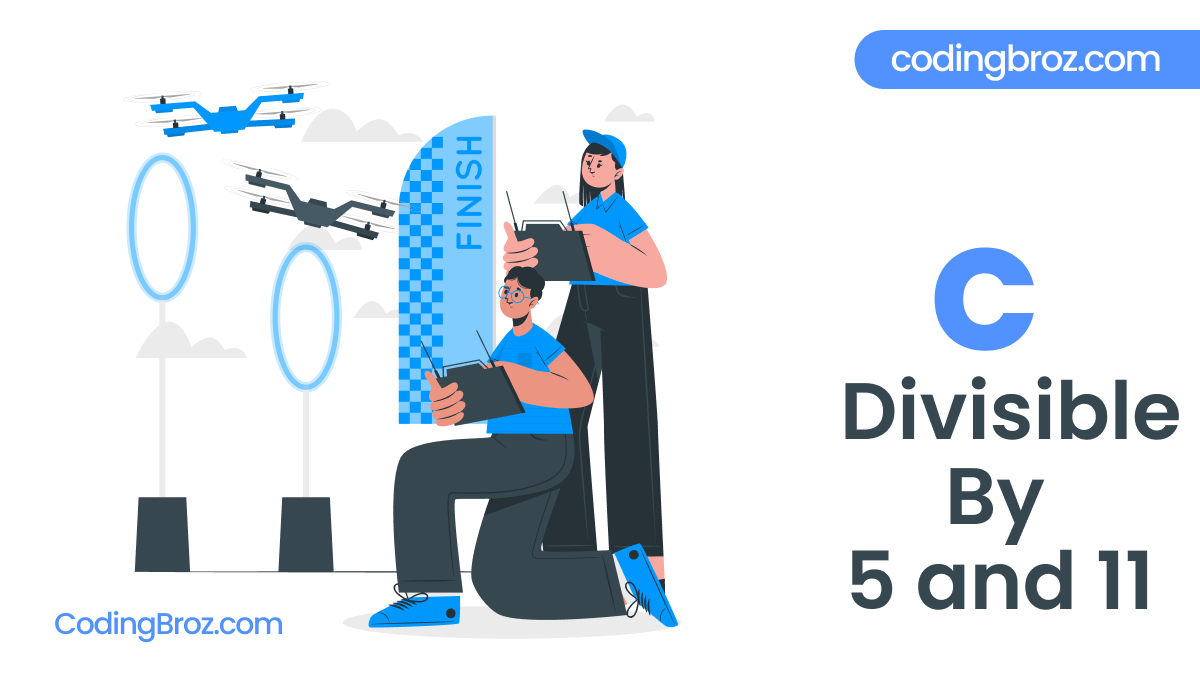
We will be using three different approaches to write the program. The three approaches are as follow:-
- Using If-Else Statement
- Using Switch Case
- Using Conditional Operator
So, without further ado, let’s begin the tutorial.
C Program to Check Whether a Number is Divisible by 5 and 11
// C Program To Check Whether a Number is Divisible by 5 and 11 #include <stdio.h> int main(){ int num; // Asking for Input printf("Enter an number: "); scanf("%d", &num); if ((num % 5 == 0) && (num % 11 == 0)){ printf("%d is divisible by both 5 and 11.", num); } else{ printf("%d is not divisible by both 5 and 11.", num); } return 0; }
Output 1
Enter an number: 55
55 is divisible by both 5 and 11.
Output 2
Enter an number: 44
44 is not divisible by both 5 and 11.
C Program to Check Whether a Number is Divisible by 5 and 11 Using Switch…case
// C Program To Check Whether a Number is Divisible by 5 and 11 #include <stdio.h> int main(){ int num; // Asking for Input printf("Enter an number: "); scanf("%d", &num); switch ((num % 5 == 0) && (num % 11 == 0)){ case 0: printf("%d is not divisible by both 5 and 11.", num); break; case 1: printf("%d is divisible by both 5 and 11.", num); break; } return 0; }
Output
Enter an number: 880
880 is divisible by both 5 and 11.
C Program to Check Whether a Number is Divisible by 5 and 11 Using Conditional Operator
// C Program To Check Whether a Number is Divisible by 5 and 11 #include <stdio.h> int main(){ int num; // Asking for Input printf("Enter an number: "); scanf("%d", &num); ((num % 5 == 0) && (num % 11 == 0)) ? printf("%d is divisible by both 5 and 11.", num): printf("%d is not divisible by both 5 and 11.", num); return 0; }
Output 1
Enter an number: 440
440 is divisible by both 5 and 11.
Output 2
Enter an number: 650
650 is not divisible by both 5 and 11.
Conclusion
I hope after going through this post, you understand how to check whether a number is divisible by 5 and 11 using C Programming language.
If you have any doubt regarding the problem, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: