In this post, we will learn how to find the average of two numbers using C Programming language.
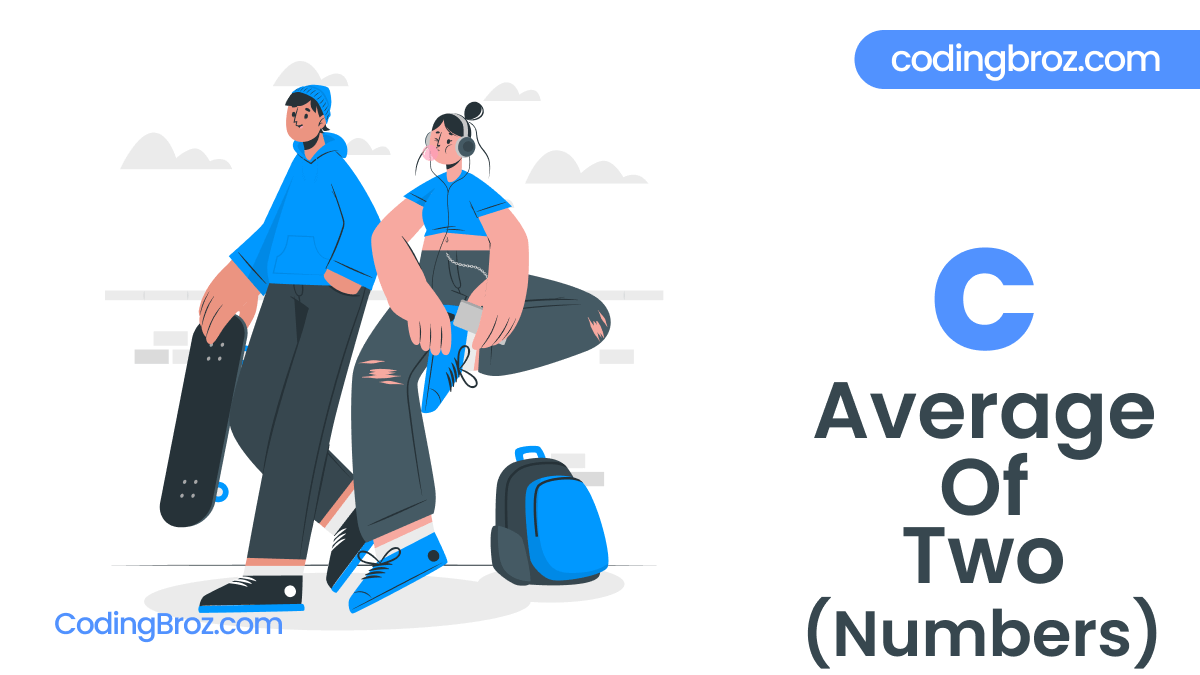
An Average is a number that is calculated by adding quantities together and then dividing the total by the number of quantities.
For Example: The Average of 7, 8, 12 is 9.
Average = Total Sum / Total no. of Quantities = (7 + 8 + 12) / 3 = 9
We will be using two different approaches to find the average. First one by using a standard method and second one by using a user defined function.
So, Without further ado, let’s begin the tutorial.
C Program To Find The Average of Two Numbers
// C Program To Find the Average of Two Numbers #include <stdio.h> int main(){ int a, b; float average; // Asking for Input printf("Enter First Number: "); scanf("%d", &a); printf("Enter Second Number: "); scanf("%d", &b); average = (float)(a + b) / 2; printf("The Average of %d and %d is %.2f.",a, b, average); return 0; }
Output
Enter First Number: 5
Enter Second Number: 8
The Average of 5 and 8 is 6.50.
How Does This Program Work ?
int a, b;
float average;
In this program, we have declared two int data type variables and one float data type variable named as a, b and average respectively.
// Asking for Input
printf("Enter First Number: ");
scanf("%d", &a);
printf("Enter Second Number: ");
scanf("%d", &b);
Then, the user is asked to enter two numbers. The value of the first and second number will get stored in a and b named variables.
average = (float)(a + b) / 2;
The average of two numbers is calculated by adding those two numbers and dividing the sum by total no. of quantities.
printf("The Average of %d and %d is %.2f.",a, b, average);
Finally, the average is displayed to the screen using printf() function. Here, we have used %.2f format because we want to display the result till 2 decimal places only.
C Program To Find The Average of Two Numbers Using Function
// C Program To Find The Average of Two Numbers Using Function #include <stdio.h> float average(int x, int y){ return (float)(x + y) / 2; } int main(){ int a, b; float result; // Asking for Input printf("Enter First Number: "); scanf("%d", &a); printf("Enter Second Number: "); scanf("%d", &b); result = average(a, b); printf("The Average of %d and %d is %.2f", a, b, result); return 0; }
Output
Enter First Number: 17
Enter Second Number: 18
The Average of 17 and 18 is 17.50
float average(int x, int y){
return (float)(x + y) / 2;
}
In this program, we have declared a custom function named as average which returns the average of two numbers.
Then, we call this function in the main function whenever we need it. It calculates and displays the average of any two numbers entered by the user.
Conclusion
I hope after going through this post, you understand how to find the average of two numbers using C Programming language.
If you have any doubt related to the program, feel free to contact us in the comment section. We will be delighted to help you.