In this post, we will learn how to find sum of even and odd numbers using the C Programming language.
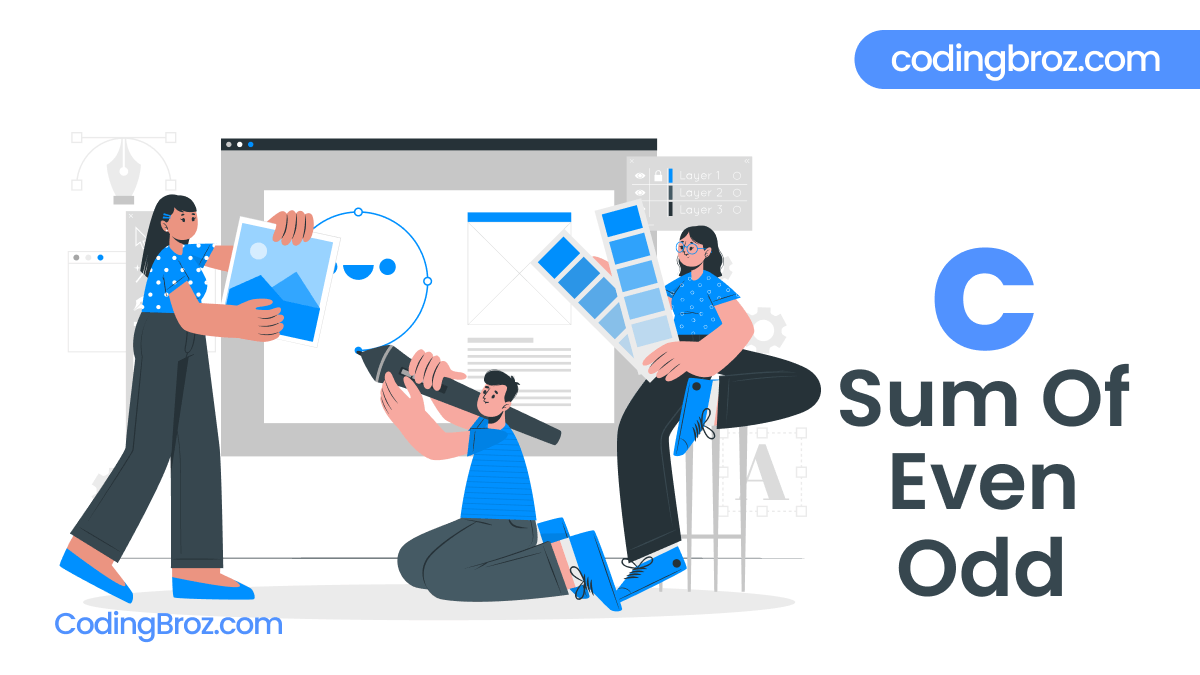
As we know, whole numbers which are exactly divisible by 2 are known as Even Numbers. For example: 2, 4, 12, 16, 256, . . . so on.
Similarly, whole numbers which are not exactly divisible by 2 are known as Odd Numbers. For example: 3, 5, 7, 13, 15, 247, . . . so on.
We will be calculating the sum of both even and odd numbers lying in a given range by using the divisibility logic.
So, without further ado, let’s begin this tutorial.
C Program to find Sum of Even and Odd Numbers
// C Program To Find Sum of Even and Odd Numbers #include <stdio.h> int main(){ int i, num, even_sum = 0, odd_sum = 0; // Asking for Input printf("Enter the Maximum Value: "); scanf("%d", &num); for (i = 1; i <= num; i++){ if (i % 2 == 0){ even_sum = even_sum + i; } else{ odd_sum = odd_sum + i; } } printf("The Sum of all Even Numbers up to %d is %d.\n", num, even_sum); printf("The Sum of all Odd Numbers up to %d is %d.", num, odd_sum); return 0; }
Output
Enter the Maximum Value: 100
The Sum of all Even Numbers up to 100 is 2550.
The Sum of all Odd Numbers up to 100 is 2500.
How Does This Program Work ?
int i, num, even_sum = 0, odd_sum = 0;
In this program, we have declared four int data type variables named i, num, even_sum and odd_sum.
// Asking for Input
printf("Enter the Maximum Value: ");
scanf("%d", &num);
Then, the user is asked to enter the value of the maximum number.
for (i = 1; i <= num; i++){
if (i % 2 == 0){
even_sum = even_sum + i;
}
else{
odd_sum = odd_sum + i;
}
}
We have used for loop here, because for loop will make sure that the number lies between 1 and Maximum Value.
If the number is divisible by 2, then it satisfies the first condition, hence it is an even number. And we add the value of i to even_sum.
If the condition is false, then it means the number is an odd number. In this case, we add the value of i to odd_sum.
printf("The Sum of all Even Numbers up to %d is %d.\n", num, even_sum);
printf("The Sum of all Odd Numbers up to %d is %d.", num, odd_sum);
Finally, the sum of even and odd numbers is printed to the screen using printf() function. Here, %d format specifier indicates int data type.
C Program To Find The Sum of Even and Odd Numbers in a Given Range
// C Program To Find Sum of Even and Odd Numbers #include <stdio.h> int main(){ int i, min, max, even_sum = 0, odd_sum = 0; // Asking for Input printf("Enter the Minimum Value: "); scanf("%d", &min); printf("Enter the Maximum Value: "); scanf("%d", &max); for (i = min; i <= max; i++){ if (i % 2 == 0){ even_sum = even_sum + i; } else{ odd_sum = odd_sum + i; } } printf("The Sum of all Even Numbers between %d and %d is %d.\n", min, max, even_sum); printf("The Sum of all Odd Numbers between %d and %d is %d.", min, max, odd_sum); return 0; }
Output
Enter the Minimum Value: 10
Enter the Maximum Value: 50
The Sum of all Even Numbers between 10 and 50 is 630.
The Sum of all Odd Numbers between 10 and 50 is 600.
Conclusion
I hope after going through this post, you understand how to find the sum of even and odd Numbers Using C Programming language.
If you have any doubt regarding the problem, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: