Hello coders, today we are going to solve Validating and Parsing Email Addresses HackerRank Solution in Python.
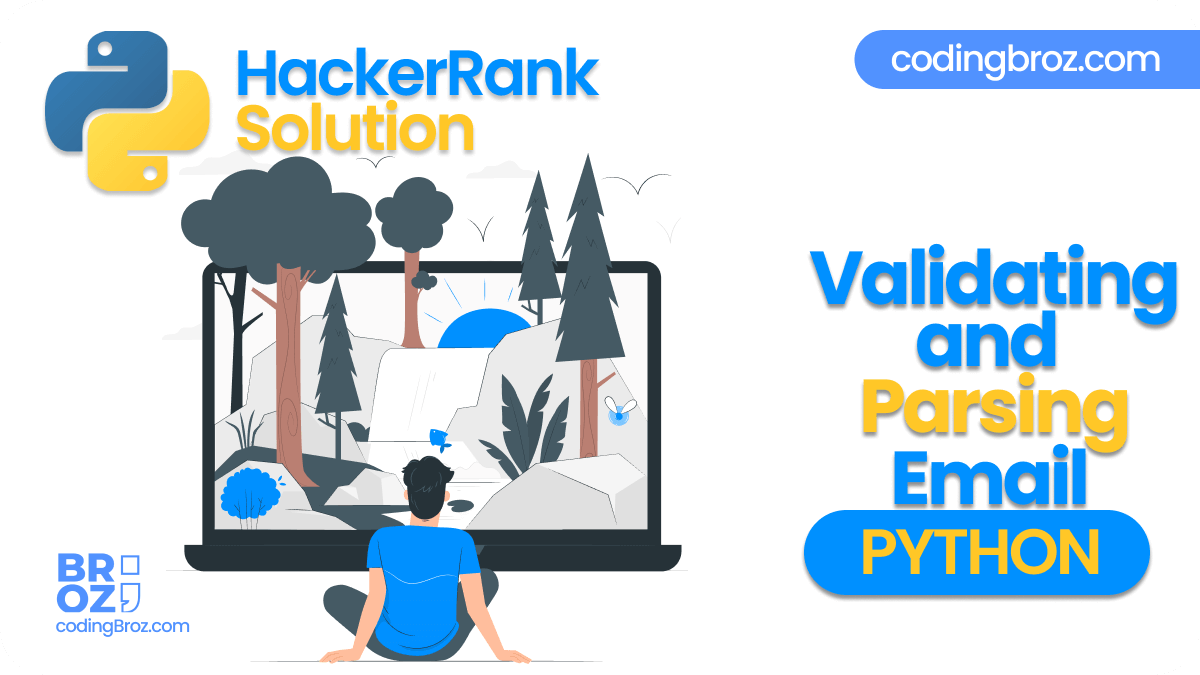
Task
A valid email address meets the following criteria:
- It’s composed of a username, domain name, and extension assembled in this format:
[email protected]
- The username starts with an English alphabetical character, and any subsequent characters consist of one or more of the following: alphanumeric characters,
-
,.
, and_
. - The domain and extension contain only English alphabetical characters.
- The extension is 1, 2, or 3 characters in length.
Given n pairs of names and email addresses as input, print each name and email address pair having a valid email address on a new line.
Hint: Try using Email.utils() to complete this challenge. For example, this code:
import email.utils
print email.utils.parseaddr('DOSHI <[email protected]>')
print email.utils.formataddr(('DOSHI', '[email protected]'))
produces this output:
('DOSHI', '[email protected]')
DOSHI <[email protected]>
Input Format
The first line contains a single integer, n, denoting the number of email address.
Each line i of the n subsequent lines contains a name and an email address as two space-separated values following this format:
name <[email protected]>
Constraints
- 0 < n < 100
Output Format
Print the space-separated name and email address pairs containing valid email addresses only. Each pair must be printed on a new line in the following format:
name <[email protected]>
You must print each valid email address in the same order as it was received as input.
Sample Input
2
DEXTER <[email protected]>
VIRUS <virus!@variable.:p>
Sample Output
DEXTER <[email protected]>
Explanation
[email protected] is a valid email address, so we print the name and email address pair received as input on a new line.
virus!@variable.:p is not a valid email address because the username contains an exclamation point (!
) and the extension contains a colon (:
). As this email is not valid, we print nothing.
Solution – Validating and Parsing Email Addresses in Python
# Enter your code here. Read input from STDIN. Print output to STDOUT import re pattern = r'^<[A-Za-z](\w|-|\.|_)+@[A-Za-z]+\.[A-Za-z]{1,3}>$' for _ in range(int(input())): name, email = input().split(' ') if re.match(pattern, email): print(name, email)
Disclaimer: The above Problem (Validating and Parsing Email Addresses) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.