Hello coders, today we are going to solve Utopian Tree HackerRank Solution which is a Part of HackerRank Algorithm Series.
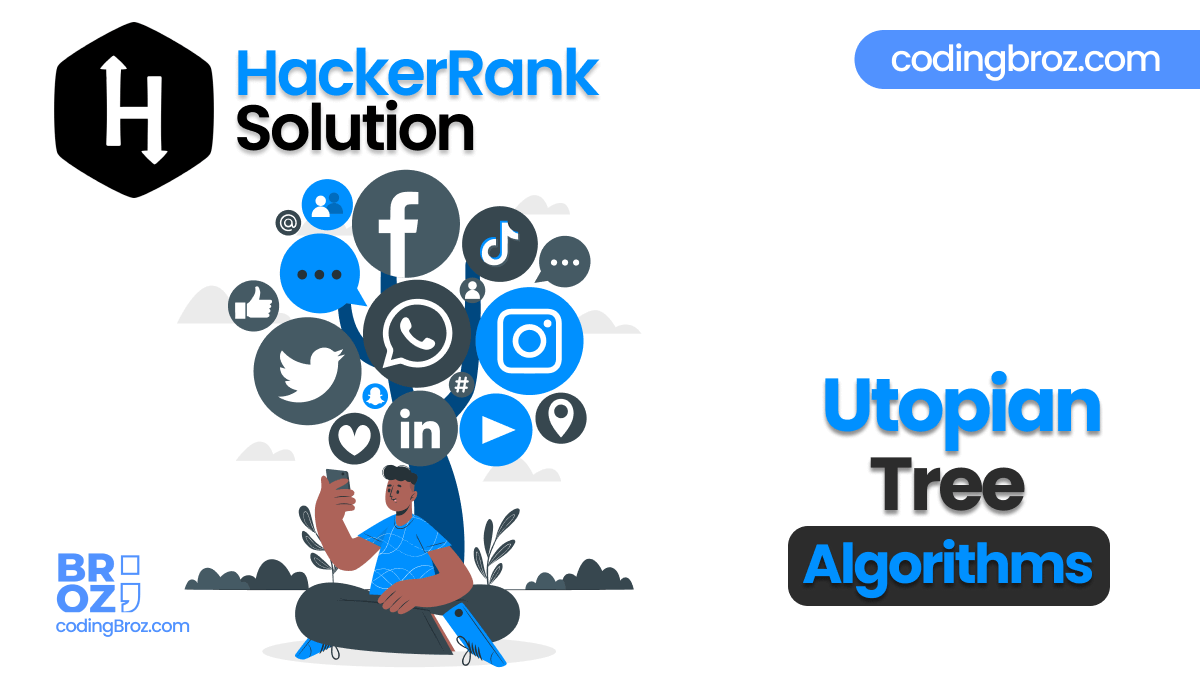
Task
The Utopian Tree goes through 2 cycles of growth every year. Each spring, it doubles in height. Each summer, its height increases by 1 meter.
A Utopian Tree sapling with a height of 1 meter is planted at the onset of spring. How tall will the tree be after n growth cycles?
For example, if the number of growth cycles is n = 5, the calculations are as follows:
Period Height
0 1
1 2
2 3
3 6
4 7
5 14
Function Description
Complete the utopianTree function in the editor below.
utopianTree has the following parameter(s):
- int n: the number of growth cycles to simulate
Returns
- int: the height of the tree after the given number of cycles
Input Format
The first line contains an integer, t, the number of test cases.
t subsequent lines each contain an integer, n, the number of cycles for that test case.
Constraints
- 1 <= t <= 10
- 0 <= n <= 60
Sample Input
3
0
1
4
Sample Output
1
2
7
Explanation
There are 3 test cases.
In the first case (n = 0), the initial height (H = 1) of the tree remains unchanged.
In the second case (n = 1), the tree doubles in height and is 2 meters tall after the spring cycle.
In the third case (n = 4), the tree doubles its height in spring (n = 1, H = 2), then grows a meter in summer (n = 2, H = 3), then doubles after the next spring (n = 3, H = 6), and grows another meter after summer (n = 4, H = 7). Thus, at the end of 4 cycles, its height is 7 meters.
Solution – Utopian Tree
C++
#include <cmath> #include <cstdio> #include <vector> #include <iostream> #include <algorithm> using namespace std; int main() { int T; cin >> T; for(int t = 0; t<T; t++){ int N; cin >> N; int ht = 1; for(int i=0;i<N;i++){ if(i%2==0) ht*=2; else ht++; } cout << ht << endl; } return 0; }
Python
import sys if __name__ == '__main__': T = int(sys.stdin.readline()) for _ in range(T): N = int(sys.stdin.readline()) height = 1 for i in range(N): if i % 2 == 0: height *= 2 else: height += 1 print(height)
Java
import java.util.Scanner; import java.util.Vector; public class Solution { public static void main(String[] args) { Scanner stdin = new Scanner(System.in); int numCases = stdin.nextInt(); Vector<Long> cache = new Vector<>(); cache.add(1L); for (int i = 0; i < numCases; i++) { int numCycles = stdin.nextInt(); while(cache.size() <= numCycles) { if (cache.size() % 2 == 0) { cache.add(cache.lastElement() + 1); } else { cache.add(cache.lastElement() * 2); } } System.out.println(cache.get(numCycles)); } } }
Disclaimer: The above Problem (Utopian Tree) is generated by Hacker Rank but the Solution is provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.