Hello coders, today we are going to solve Small Triangles, Large Triangles HackerRank Solution in C.
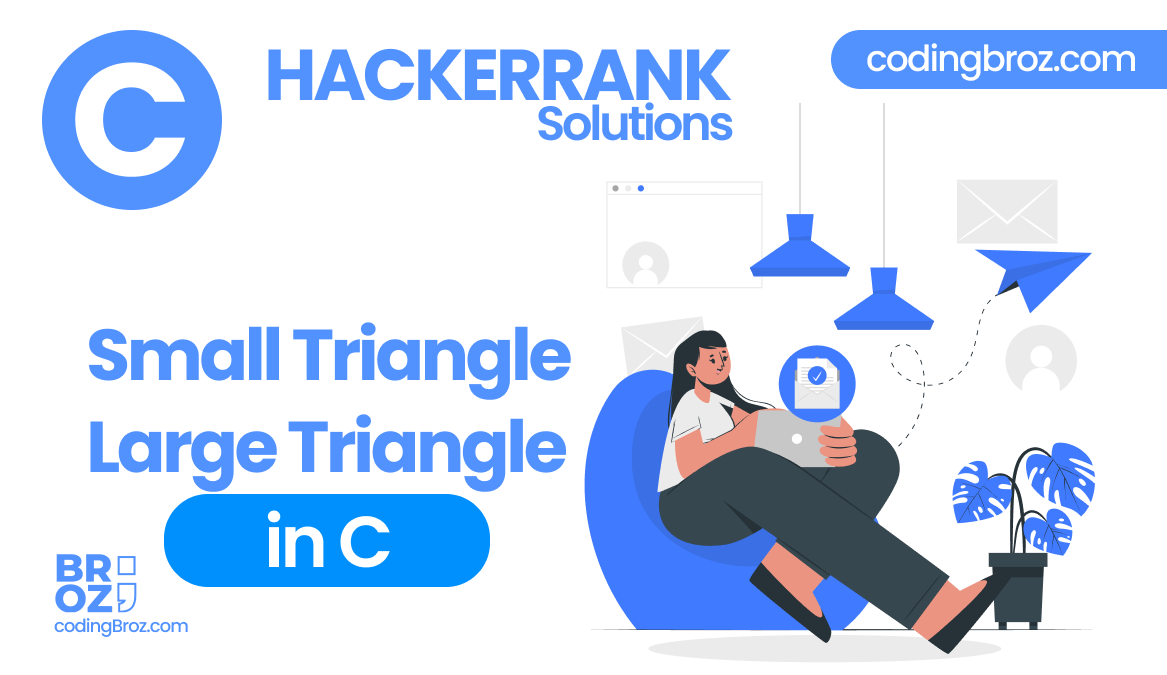
Problem
You are given n triangles, specifically, their sides ai, bi and ci. Print them in the same style but sorted by their areas from the smallest one to the largest one. It is guaranteed that all the areas are different.
The best way to calculate a area of a triangle with sides a, b and c is Heron’s formula:
s = p x [(p -a) x (p -b) x (p – c)]^1/2 where p = a + b + c / 2.
Input Format
The first line of each test file contains a single integer n. n lines follow with three space-separated integers, ai, bi and ci.
Constraints
- 1 <= n <= 100
- 1 <= ai, bi, ci <= 70
- ai + bi > ci, ai + ci > bi and bi + ci > ai.
Output Format
Print exactly n lines. On each line print 3 space-separated integers, the ai, bi and ci of the corresponding triangle.
Sample Input 0
3 7 24 25 5 12 13 3 4 5
Sample Output 0
3 4 5 5 12 13 7 24 25
Explanation 0
The square of the first triangle is 84. The square of the second triangle is 30. The square of the third triangle is 6. So the sorted order is the reverse one.
Solution – Small Triangles, Large Triangles in C
C
void sort_by_area(triangle* tr, int n) { /** * Sort an array a of the length n */ int *p=malloc(n*sizeof(int)); //create array of size n to store "volumes" for(int i=0;i<n;i++) { float a=(tr[i].a+tr[i].b+tr[i].c)/2.0; //use 2.0 compulsary int/int gives int, int/float gives float p[i]=(a*(a-tr[i].a)*(a-tr[i].b)*(a-tr[i].c)); //formula without sqrt as areas are different guarenteed //because sqrt dosent work well with float values } //bubble sort for(int i=0;i<n;i++) { for(int j=0;j<n-i-1;j++) { if(p[j]>p[j+1]) { int temp=p[j]; p[j]=p[j+1]; p[j+1]=temp; //swapping array of areas in ascending //and simuntaneously the structure contents temp=tr[j].a; tr[j].a=tr[j+1].a; tr[j+1].a=temp; temp=tr[j].b; tr[j].b=tr[j+1].b; tr[j+1].b=temp; temp=tr[j].c; tr[j].c=tr[j+1].c; tr[j+1].c=temp; } } } }
Disclaimer: The above Problem (Small Triangles, Large Triangles) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.