Hello coders, Today we are going to solve this amazing string problem which also test our knowledge in binary arithmetic. We need to add two binary strings.
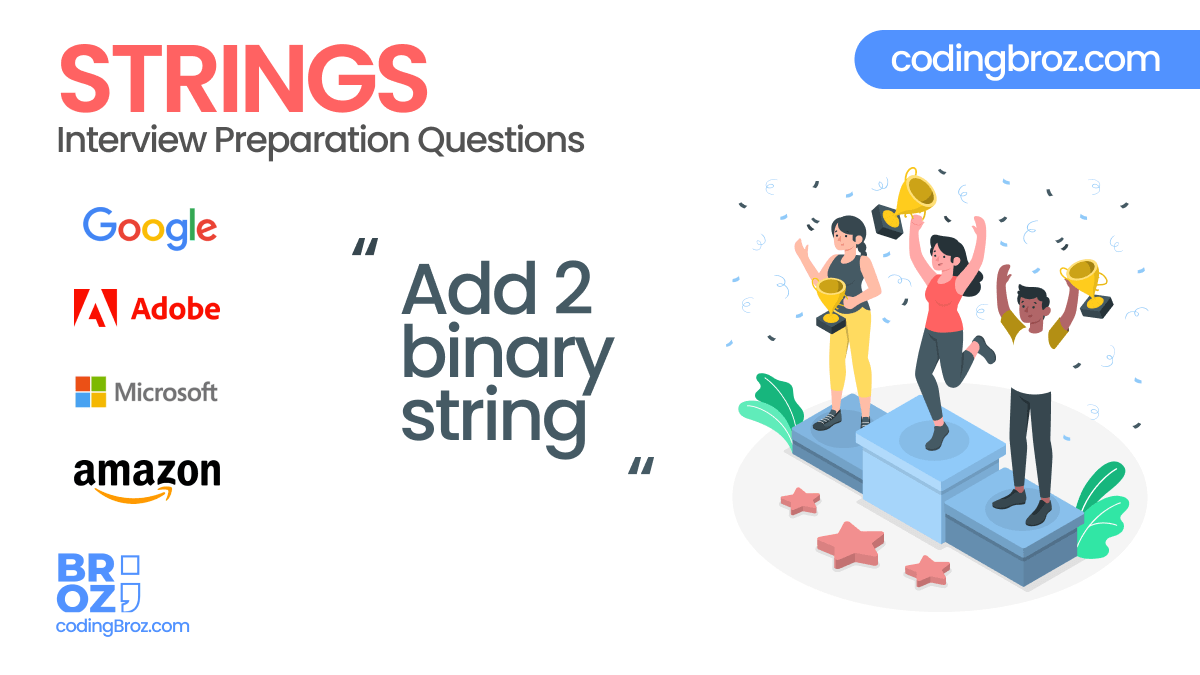
Task
Given two strings with binary number, we have to find the result obtained by adding those two binary strings and return the result as a binary string.
Binary numbers are those numbers which are expressed either as 0 or 1. While adding 2 binary numbers there is binary addition rule which is to be taken care of.
0+0 → 0
0+1 → 1
1+0 → 1
1+1 → 0, carry 1
Input
str1 = {“11”}, str2 = {“1”}
Output
“100”
Input
str1 = {“110”}, str2 = {“1”}
Output
“111”
Program to add 2 binary string in Java
public class AddBinaryStrings { /** * Main method * @param args * @throws Exception */ public static void main(String[] args) throws Exception { System.out.println(new AddBinaryStrings().addBinary("1111110101010101010", "1111010101010101010101010101010101")); } private String addBinary(String s1, String s2) { String first, second; if(s1.length() >= s2.length()) { first = s1; second = s2; } else { first = s2; second = s1; } int carry = 0; StringBuilder result = new StringBuilder(); for(int i = first.length() - 1, j = second.length() - 1; i >= 0; i--, j--) { int one = ('0' - first.charAt(i)) * -1; int two = 0; if(j >= 0) { two = ('0' - second.charAt(j)) * -1; } result.append((one + two + carry) % 2); carry = (one + two + carry) / 2; } if(carry > 0) result.append(carry); return result.reverse().toString(); } }