Hello coders, today we are going to solve Mod Divmod HackerRank Solution in Python.
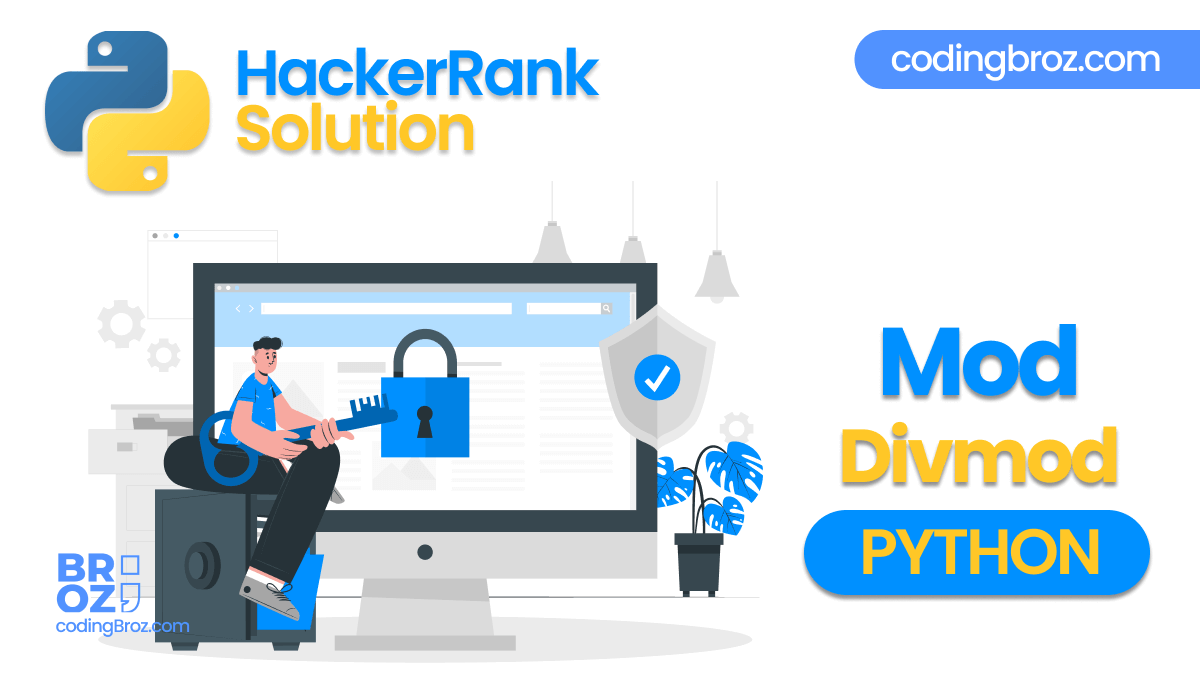
Objective
One of the built-in functions of Python is divmod, which takes two arguments and and returns a tuple containing the quotient of a/b first and then the remainder a.
For example:
>>> print divmod(177,10)
(17, 7)
Here, the integer division is 177/10 => 17
and the modulo operator is 177%10 => 7
.
Task
Read in two integers, a and b, and print three lines.
The first line is the integer division a//b (While using Python2 remember to import division
from __future__
).
The second line is the result of the modulo operator: a%b.
The third line prints the divmod of a and b.
Input Format
The first line contains the first integer, a, and the second line contains the second integer, b.
Output Format
Print the result as described above.
Sample Input
177
10
Sample Output
17
7
(17, 7)
Solution – Mod Divmod in Python
# Enter your code here. Read input from STDIN. Print output to STDOUT A = int(input()) B = int(input()) print(A//B) print(A%B) print(divmod(A, B))
Disclaimer: The above Problem (Mod Divmod) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.