In this post, we will learn how to find the average of N numbers using JavaScript.
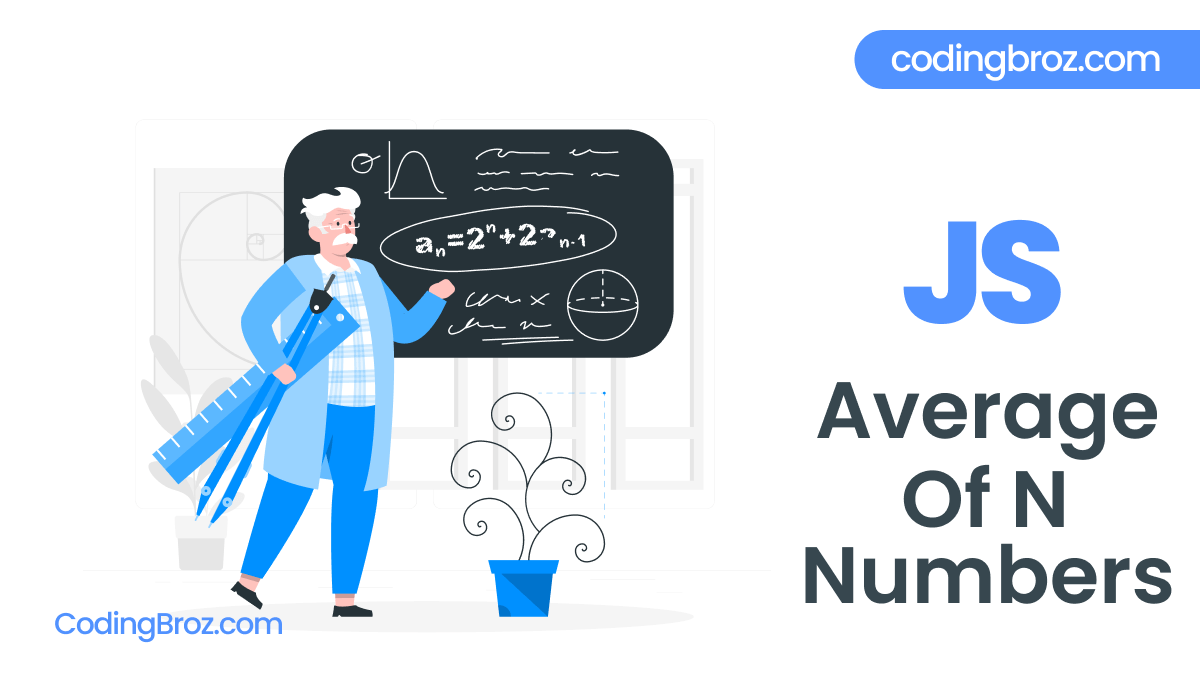
This program asks the user to enter the first and last digit, then it computes the average of N numbers lying between them.
So, without further ado, let’s begin this tutorial.
JavaScript Program To Find Average of N Numbers
var start = parseInt(prompt("Enter the Starting Number")); var end = parseInt(prompt("Enter the Ending Number")); var total = 0; var count = (end - start) + 1 var average = 0; console.log("Average of Numbers between " + start + " and " + end + " is: " ); for (start = start; start <= end; start++) total = total + start; average = total / count; console.log("Total Sum: " + total); console.log("No. of elements: " + count); console.log("Average: " + average);
Output
Average of Numbers between 1 and 5 is:
Total Sum: 15
No. of elements: 5
Average: 3
How Does This Program Work ?
var start = parseInt(prompt("Enter the Starting Number"));
var end = parseInt(prompt("Enter the Ending Number"));
In this program, the user is asked to enter the first and last digit of the series.
var total = 0;
var count = (end - start) + 1
var average = 0;
We declare 3 three int data type variables. total will store the total sum, count will store the value of total number of elements and average will store the average of numbers.
for (start = start; start <= end; start++)
total = total + start;
We calculate the sum of the total numbers lying between start and end using a for loop.
average = total / count;
Then, average is calculated by dividing the total sum / number of elements.
console.log("Average: " + average);
Finally, the average of total numbers is printed using the console.log() function.
Conclusion
I hope after going through this post, you understand how to find the average of N numbers using JavaScript.
If you have any doubt regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: