Hello coders, today we are going to solve Java Loops I HackerRank Solution.
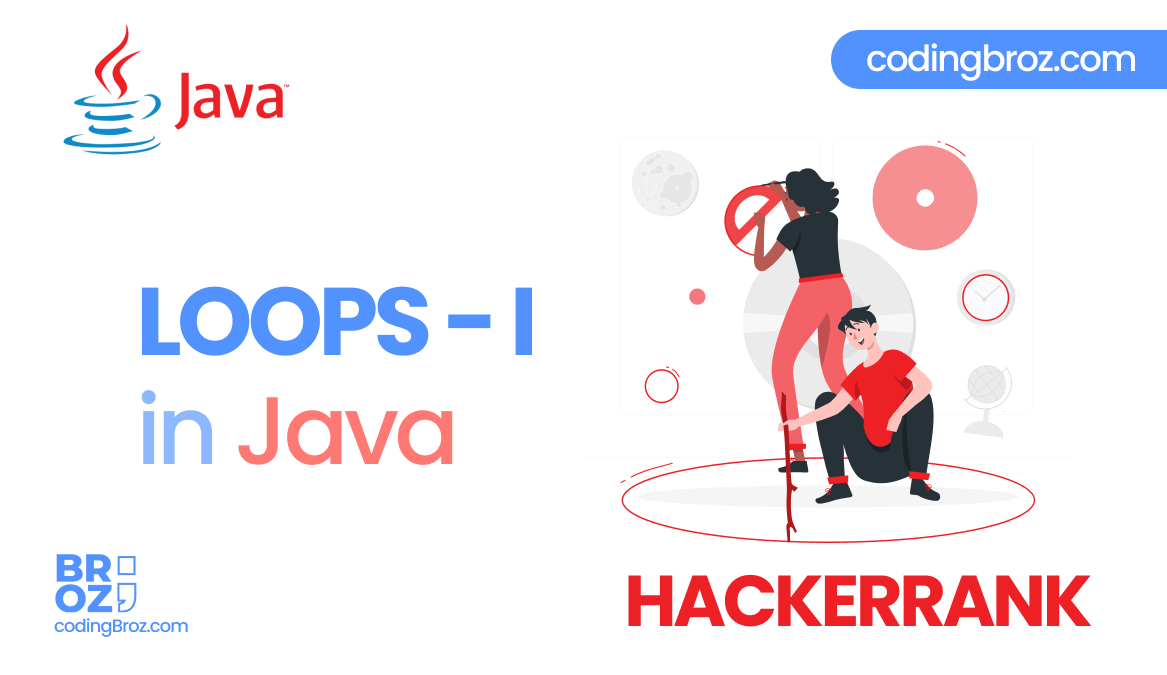
Problem
In this challenge, we’re going to use loops to help us do some simple math.
Task
Given an integer, N, print its first 10 multiples. Each multiple N X i (where 1<=i<=10) should be printed on a new line in the form: N x i = result.
Input Format
A single integer, N.
Constraints
2 <= N <= 20 .
Output Format
Print 10 lines of output; each line i (where 1 <= i <= 10) contains the result of N X i in the form:
N x i = result.
Sample input
2
Sample output
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
Solution – Java Loops 1
import java.io.*; import java.math.*; import java.security.*; import java.text.*; import java.util.*; import java.util.concurrent.*; import java.util.regex.*; public class Solution { private static final Scanner scanner = new Scanner(System.in); public static void main(String[] args) { int N = scanner.nextInt(); scanner.skip("(\r\n|[\n\r\u2028\u2029\u0085])?"); if(N>1 && N<=20) { for(int i=1 ; i<=10 ; i++) { int mul=N*i; System.out.println(N+" x "+i+" = "+mul); } } scanner.close(); } }
Disclaimer: The above Problem ( Java Loops 1 ) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.