Hello Coderz ! Welcome to CodingBroz where we make you understand programming concepts and help in making programming easy and fun to you.
Today, I will be going to tell you about a Basic Java program on How to take input from users in Java?
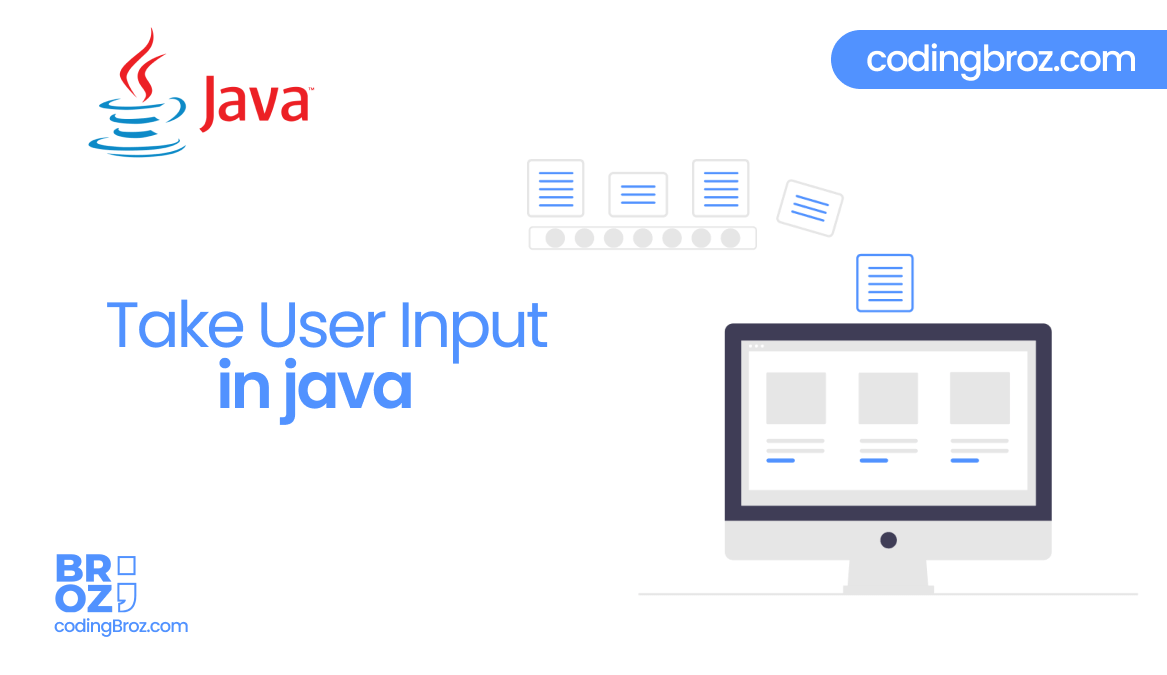
Java Scanner Class
In Java, Scanner Class allows us to take input from the console. Scanner Class is available in the java.util package.
It is used to read the input from the console by the user. It reads all the primitive data types like int, double, float, boolean, char, e.t.c. It is the easiest way to take input from users in Java.
Syntax : To declare scanner class
Scanner scan = new Scanner(System.in);
This code above calls the constructor of the Scanner class and takes System.in as an argument. We need to import the java.util package to use the Scanner class.
Methods in Scanner class
- nextInt() – It is used to take Integer datatype as an input.
- nextDouble() – It is used to take a Double datatype as an input.
- nextFloat() – It is used to take Float datatype as an input.
- nextByte() – It is used to take the next input as a byte.
- nextShort()- It is used to take the next input as short data type.
- nextLine() – It takes the input of the whole line as a String.
- nextBoolean() – It is used to take Boolean datatype as its input.
- nextBigInteger() – It is used to scan the next token as a BigInteger.
- nextBigDouble() – It is used to scan the next token as a BigDouble value.
Now, let’s see how to use the Scanner class and its methods to take input from the users in Java.
How to input Integer in Java ?
Let’s see the code to input Integer in Java from user :
public static void main(String[] args) { Scanner sc= new Scanner(System.in); //System.in is a standard input stream System.out.print("Enter a string: "); int i = sc.nextInt(); //reads Integer System.out.print("You have entered: "+i); }
How to input String in Java ?
Let’s see the code to input Integer in Java from user :
public static void main(String[] args) { Scanner sc= new Scanner(System.in); //System.in is a standard input stream System.out.print("Enter a string: "); String str = sc.nextLine(); //reads String System.out.print("You have entered: "+str); }
Important Point #1
Whenever you need to take input of a String after an Integer or a double value then you must write a dummy sc.nextLine() line and then take the input of String. Otherwise you won’t be able to take the input.
For example see this code :
public static void main(String[] args) { Scanner sc= new Scanner(System.in); //System.in is a standard input stream int i = sc.nextInt(); //reads Integer String s = sc.nextLine(); // here the code will fail and not be able to take any input }
Just try the above in your IDE and notice the flaw in the above code and experience it yourself.
So, why does this happen that you are not able to take your desired String as an input just after taking input of an Integer/ a double ?
This is because when you write integer input in the console and press the enter key. The enter key itself is taken as an input there because the enter key is a special character.
So, to avoid this you need to do this instead :
public static void main(String[] args) { Scanner sc= new Scanner(System.in); //System.in is a standard input stream int i = sc.nextInt(); //reads Integer sc.next() // dummy line String s = sc.nextLine(); // here the code will fail and not be able to take any input }
Now, your code is good to go. The above problem occurs only when string is taken as an input after taking input of any numeric value.
Broz Who Code
CodingBroz