Hello coders, today we are going to solve Day 9: Recursion 3 HackerRank Solution in C, C++ and Java.
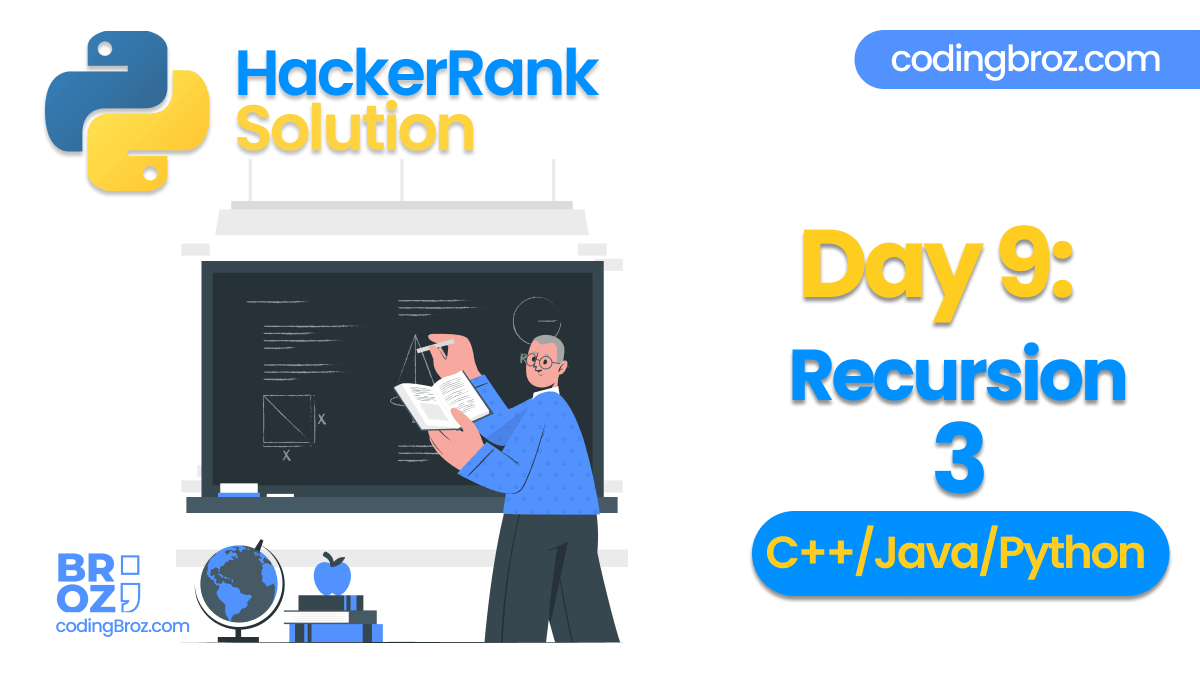
Objective
Today, we are learning about an algorithmic concept called recursion.
Function Description
Complete the factorial function in the editor below. Be sure to use recursion.
factorial has the following paramter:
- int n: an integer
Returns
- int: the factorial of n
Note: If you fail to use recursion or fail to name your recursive function factorial or Factorial, you will get a score of 0.
Input Format
A single integer, n (the argument to pass to factorial).
Constraints
- 2 <= n <= 12
- Your submission must contain a recursive function named factorial.
Sample Input
3
Sample Output
6
Explanation
Consider the following steps. After the recursive calls from step 1 to 3, results are accumulated from step 3 to 1.
- factorial(3) = 3 x factorial(2) = 3 x 2 = 6
- factorial(2) = 2 x factorial(2) = 2 x 1 = 2
- factorial(1) = 1
Solution – Day 9: Recursion 3
C
#include <stdio.h> int factorial(int n){ return (n == 1) ? 1 : n * factorial(n - 1); }; int main() { int n; scanf("%d", &n); printf("%d", factorial(n)); return 0; }
C++
#include <bits/stdc++.h> using namespace std; // Complete the factorial function below. int factorial(int n) { if(n<=1) { return 1; } else{ return n*factorial(n-1); } } int main() { ofstream fout(getenv("OUTPUT_PATH")); int n; cin >> n; cin.ignore(numeric_limits<streamsize>::max(), '\n'); int result = factorial(n); fout << result << "\n"; fout.close(); return 0; }
Java
import java.io.*; import java.math.*; import java.security.*; import java.text.*; import java.util.*; import java.util.concurrent.*; import java.util.regex.*; public class Solution { public static final Scanner sc= new Scanner(System.in); // Complete the factorial function below. static int factorial(int n) { if(n<=1){ return 1; } else{ return n * factorial(n-1); } } public static void main(String agrs[]){ int a=sc.nextInt(); System.out.println(factorial(a)); } }
Disclaimer: The above Problem (Day 9: Recursion 3) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.