Hello coders, today we are going to solve Day 5: Loops HackerRank Solution in C++, Java and Python.
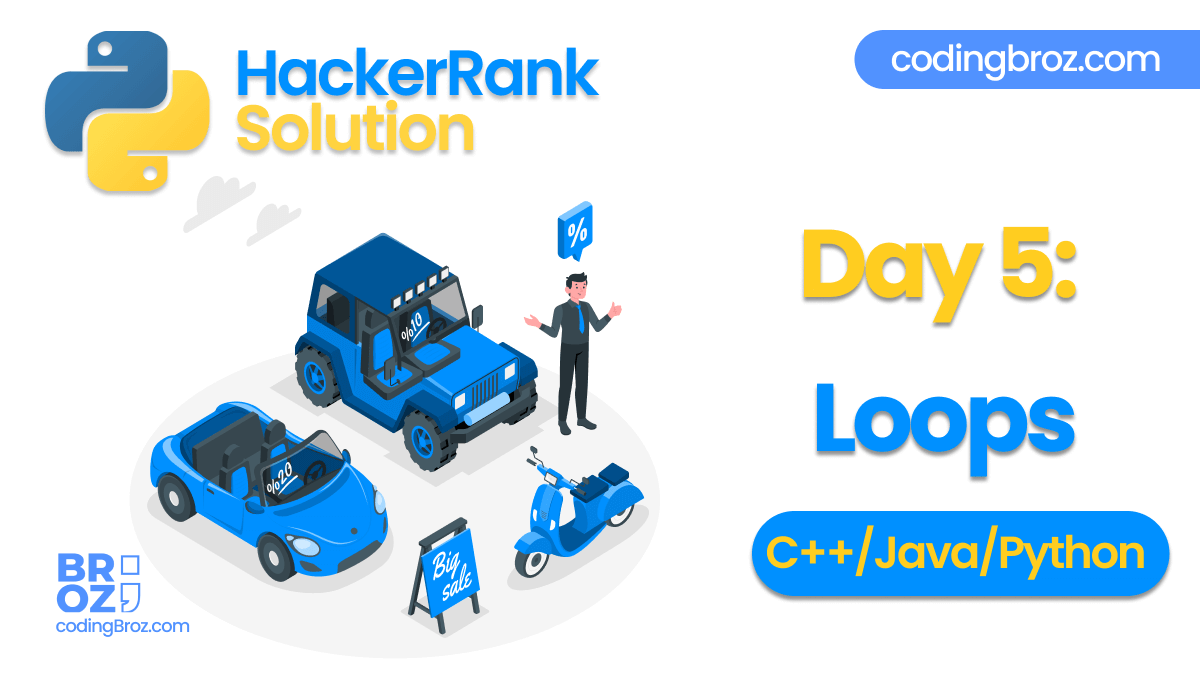
Objective
In this challenge, we will use loops to do some math.
Task
Given an integer, n, print its first 10 multiples. Each multiple n x i (where 1 <= i <= 10) should be printed on a new line in the form: n x i = result
.
Example
n = 3
The printout should look like this:
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
3 x 4 = 12
3 x 5 = 15
3 x 6 = 18
3 x 7 = 21
3 x 8 = 24
3 x 9 = 27
3 x 10 = 30
Input Format
A single integer, n.
Constraints
- 2 <= n <= 20
Output Format
Print 10 lines of output; each line i (where 1 <= i <= 10) contains the result of n x i in the form:n x i = result
.
Sample Input
2
Sample Output
2 x 1 = 2
2 x 2 = 4
2 x 3 = 6
2 x 4 = 8
2 x 5 = 10
2 x 6 = 12
2 x 7 = 14
2 x 8 = 16
2 x 9 = 18
2 x 10 = 20
Solution – Day 5: Loops
C++
#include <bits/stdc++.h> using namespace std; int main() { int n; cin >> n; cin.ignore(numeric_limits<streamsize>::max(), '\n'); if(2<=n<=20) { for (int i = 1; i <= 10; i++) { cout <<n<<" "<<"x"<<" "<<i<<" "<<"="<<" "<<(n*i)<< endl; } } return 0; }
Java
import java.io.*; import java.math.*; import java.security.*; import java.text.*; import java.util.*; import java.util.concurrent.*; import java.util.regex.*; public class Solution { private static final Scanner scanner = new Scanner(System.in); public static void main(String[] args) { int n = scanner.nextInt(); scanner.skip("(\r\n|[\n\r\u2028\u2029\u0085])?"); for (int i = 1; i <= 10; i++) { System.out.println(n + " x " + i + " = " + n * i); } scanner.close(); } }
Python
#!/bin/python3 import math import os import random import re import sys if __name__ == '__main__': n = int(input()) for i in range ( 1, 11 ): print ( n, "x", i, "=", n*i)
Disclaimer: The above Problem (Day 5: Loops) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.
Can you please explain why we have to use “x” and “=” instead of * and ==?