Hello coders, today we are going to solve Day 17: More Exceptions HackerRank Solution in C++, Java and Python.
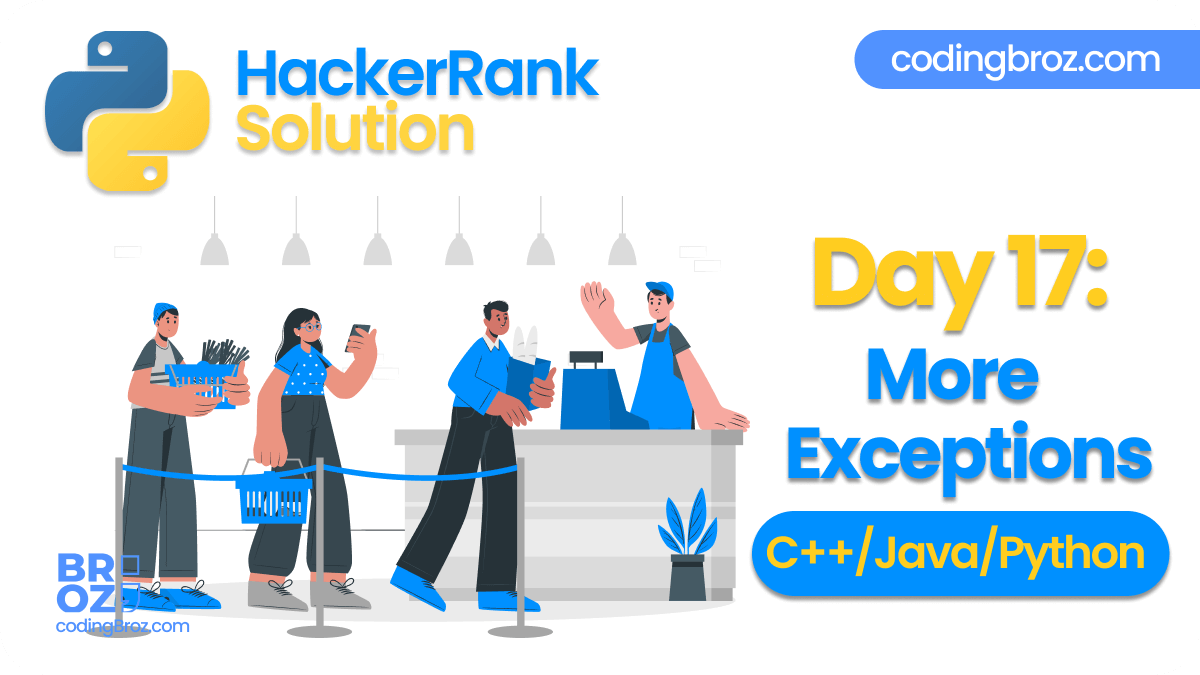
Objective
Yesterday’s challenge taught you to manage exceptional situations by using try and catch blocks. In today’s challenge, you will practice throwing and propagating an exception.
Task
Write a Calculator class with a single method: int power(int,int). The power method takes two integers, n and p, as parameters and returns the integer result of np. If either n or p is negative, then the method must throw an exception with the message: n and p should be non-negative
.
Note: Do not use an access modifier (e.g.: public) in the declaration for your Calculator class.
Input Format
Input from stdin is handled for you by the locked stub code in your editor. The first line contains an integer, , the number of test cases. Each of the T subsequent lines describes a test case in 2 space-separated integers that denote n and p, respectively.
Constraints
- No Test Case will result in overflow for correctly written code.
Output Format
Output to stdout is handled for you by the locked stub code in your editor. There are T lines of output, where each line contains the result of np as calculated by your Calculator class’ power method.
Sample Input
4
3 5
2 4
-1 -2
-1 3
Sample Output
243
16
n and p should be non-negative
n and p should be non-negative
Explanation
T = 4
T0: 3 and 5 are positive, so power returns the result of 35, which is 243.
T1: 2 and 4 are positive, so power returns the result of 24 =, which is 16.
T2: Both inputs (-1 and -2) are negative, so power throws an exception and n and p should be non-negative is printed.
T3: One of the inputs (-1) is negative, so power throws an exception and n and p should be non-negative is printed.
Solution – Day 17: More Exceptions
C++
#include <cmath> #include <iostream> #include <exception> #include <stdexcept> using namespace std; //Write your code here class Calculator { public: int power(int first, int second) { if(first < 0 || second < 0) { throw runtime_error("n and p should be non-negative"); } return pow(first, second); } }; int main() { Calculator myCalculator=Calculator(); int T,n,p; cin>>T; while(T-->0){ if(scanf("%d %d",&n,&p)==2){ try{ int ans=myCalculator.power(n,p); cout<<ans<<endl; } catch(exception& e){ cout<<e.what()<<endl; } } } }
Java
import java.util.*; import java.io.*; class Calculator { public int power(int n,int p) throws Exception{ if(n<0 || p<0){ throw new Exception("n and p should be non-negative"); } else{ return (int)Math.pow(n,p); } } } class Solution{ public static void main(String[] args) { Scanner in = new Scanner(System.in); int t = in.nextInt(); while (t-- > 0) { int n = in.nextInt(); int p = in.nextInt(); Calculator myCalculator = new Calculator(); try { int ans = myCalculator.power(n, p); System.out.println(ans); } catch (Exception e) { System.out.println(e.getMessage()); } } in.close(); } }
Python
class Calculator: def power(self, n, p): if n < 0 or p < 0: raise Exception("n and p should be non-negative") return pow(n, p) myCalculator=Calculator() T=int(input()) for i in range(T): n,p = map(int, input().split()) try: ans=myCalculator.power(n,p) print(ans) except Exception as e: print(e)
Disclaimer: The above Problem (Day 17: More Exceptions) is generated by Hacker Rank but the Solution is Provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.