Hello coders, today we are going to solve Day 1: Arithmetic Operators Hacker Rank Solution which is a part of 10 Days of JavaScript Series.
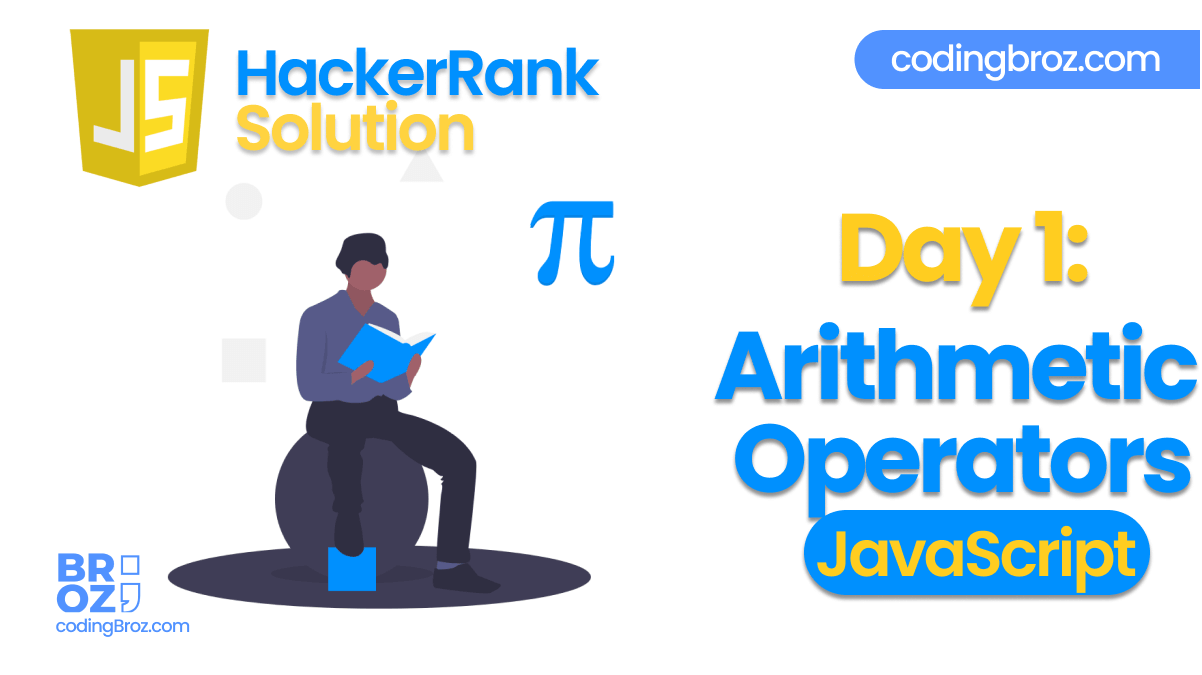
Objective
In this challenge, we practice using arithmetic operators. Check out the attached tutorial for resources.
Task
Complete the following functions in the editor below:
getArea(length, width)
: Calculate and return the area of a rectangle having sides length and width.getPerimeter(length, width)
: Calculate and return the perimeter of a rectangle having sides length and width.
The values returned by these functions are printed to stdout by locked stub code in the editor.
Input Format
getArea
Data Type | Parameter | Description |
---|---|---|
Number | length | A number denoting the length of a rectangle. |
Number | height | A number denoting the height of a rectangle. |
getPerimeter(length, height)
Data Type | Parameter | Description |
---|---|---|
Number | length | A number denoting the length of a rectangle. |
Number | height | A number denoting the height of a rectangle. |
Constraints
- 1 <= length, width <= 1000
- length and width are scaled to at most three decimal places.
Output Format
Function | Return Type | Description |
---|---|---|
getArea | Number | The area of a rectangle having sides length and width. |
getPerimeter | Number | The perimeter of a rectangle having sides length and width. |
Sample Input 0
3
4.5
Sample Output 0
13.5
15
Explanation 0
The area of the rectangle is length x width = 3 x 4.5 = 13.5.
The perimeter of the rectangle is 2 x (length + width) = 2 x (3 + 4.5) = 15.
Solution – Day 1: Arithmetic Operators
'use strict'; process.stdin.resume(); process.stdin.setEncoding('utf-8'); let inputString = ''; let currentLine = 0; process.stdin.on('data', inputStdin => { inputString += inputStdin; }); process.stdin.on('end', _ => { inputString = inputString.trim().split('\n').map(string => { return string.trim(); }); main(); }); function readLine() { return inputString[currentLine++]; } /** * Calculate the area of a rectangle. * * length: The length of the rectangle. * width: The width of the rectangle. * * Return a number denoting the rectangle's area. **/ function getArea(length, width) { let area; // Write your code here area = length * width; return area; } /** * Calculate the perimeter of a rectangle. * * length: The length of the rectangle. * width: The width of the rectangle. * * Return a number denoting the perimeter of a rectangle. **/ function getPerimeter(length, width) { let perimeter; // Write your code here perimeter = 2 *(length + width); return perimeter; }
Disclaimer: The above Problem (Arithmetic Operators) is generated by Hacker Rank but the Solution is provided by CodingBroz. This tutorial is only for Educational and Learning Purpose.
Also Read: