In this post, we will learn how to find factorial of a number using recursion in C Programming language.
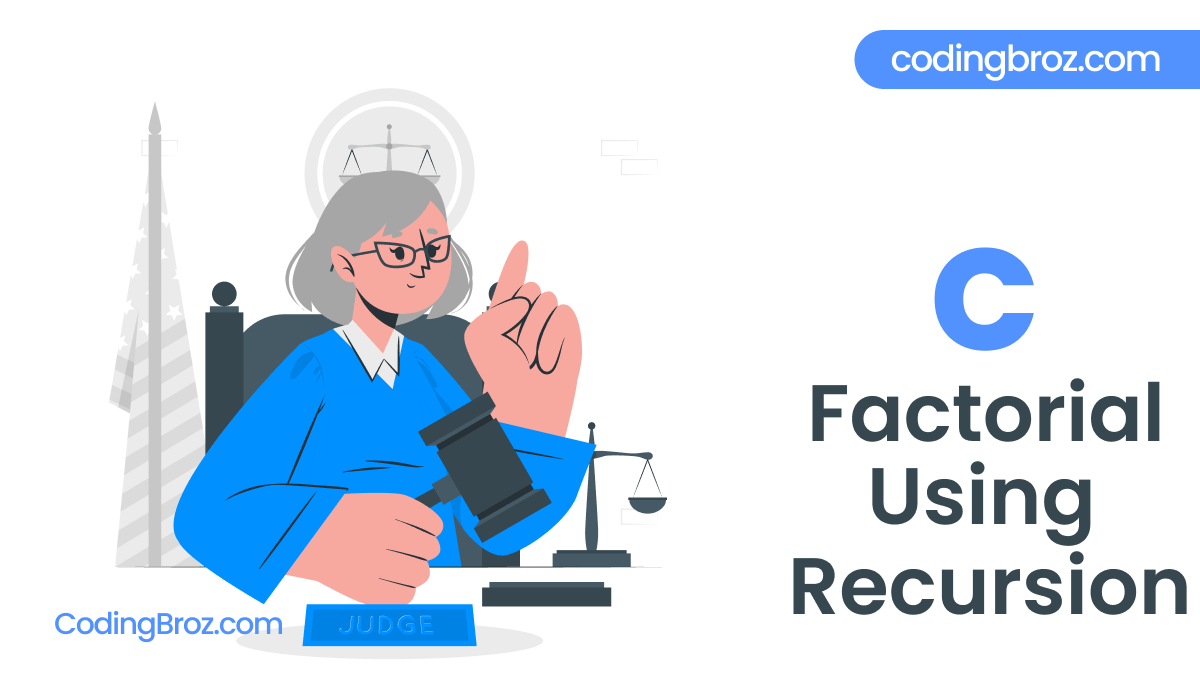
As we know, factorial of a number ‘n’ is multiplication of all integers smaller than or equal to n.
For example: The factorial of 3 is (3 x 2 x 1) = 6.
In the program, We will use a recursive function which will calculate the factorial of any number entered by the user.
So, without further ado, let’s begin this tutorial.
C Program to Find Factorial of a Number Using Recursion
// C Program To Find Factorial of a Number Using Recursion #include <stdio.h> int fact(int); int main(){ int num; // Asking for Input printf("Enter a number: "); scanf("%d", &num); printf("Factorial of %d is %d.", num, fact(num)); return 0; } int fact(int n){ if (n >= 1) return (n * fact(n - 1)); else return 1; }
Output
Enter a number: 5
Factorial of 5 is 120.
How Does This Program Work ?
int num;
In this program, we have declared an int data type variable named as num.
// Asking for Input
printf("Enter a number: ");
scanf("%d", &num);
Then, the user is asked to enter any number. The value of this number will get stored in the num named variable.
printf("Factorial of %d is %d.", num, fact(num));
We call our recursive custom function in the main function which will display the result as output.
int fact(int n){
if (n >= 1)
return (n * fact(n - 1));
else
return 1;
}
Suppose the user enters 5.
Initially, fact() is called from the main() function with 5 passed as an argument. Then, 4 is passed to fact() from the recursive call. This process continues until the value of n is less than 1. This will ultimately return 5 x 4 x 3 x 2 to the main function.
When n is less than 1, then there is no recursive call and the factorial is returned to the main() function.
Conclusion
I hope after going through this post, you understand how to find factorial of a number using recursion in C Programming language.
If you have any doubt regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: