In this post, we will check whether a year is a leap year or not using C Programming language.
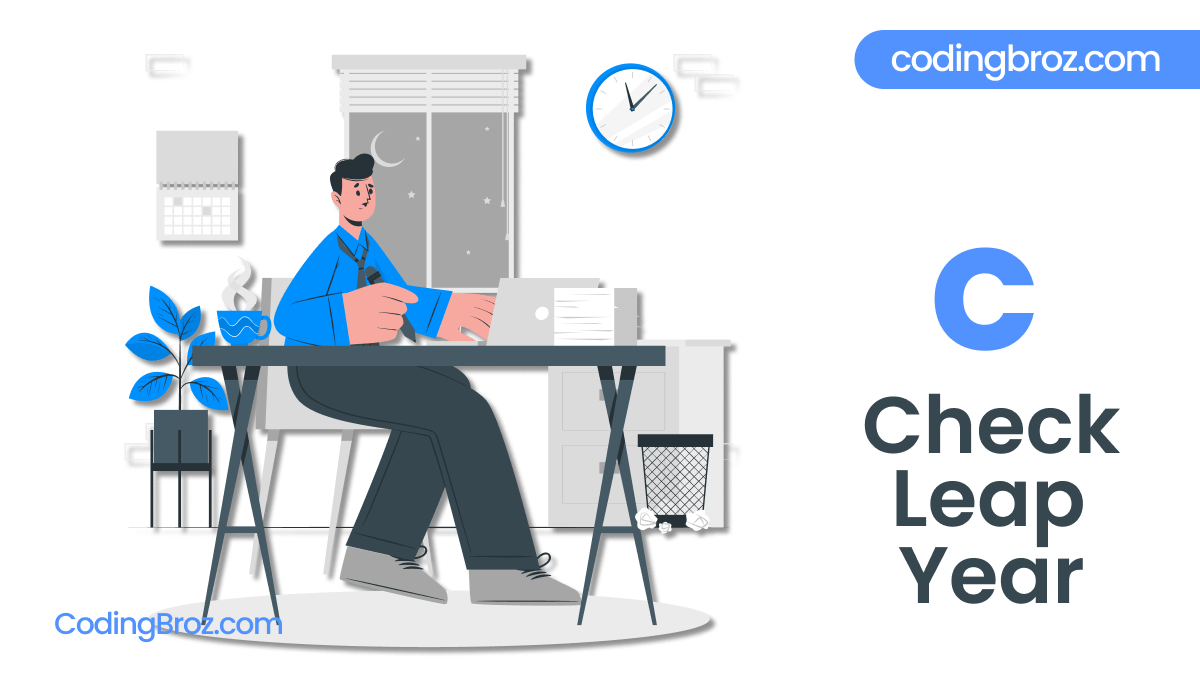
A Leap Year contains 366 days in a year. A year is a leap year if it is exactly divisible by 4 except for century years. The century years are leap years only if they are perfectly divisible by 400 .
So, without further ado, let’s begin the tutorial to check for a leap year.
C Program To Check Leap Year
// C Program To Check Leap Year #include <stdio.h> int main(){ int year; // Asking for Input printf("Enter the Year: "); scanf("%d", &year); // logic if (year % 400 == 0){ printf("%d is a leap year", year); } else if (year % 100 == 0){ printf("%d is not a leap year.", year); } else if (year % 4 == 0){ printf("%d is a leap year.", year); } else { printf("%d is not a leap year.", year); } return 0; }
Output 1
Enter the Year: 1944
1944 is a leap year.
Output 2
Enter the Year: 2026
2026 is not a leap year.
How Does This Program Work ?
int year;
Here in this program, we have declared an int variable named as year.
// Asking for Input
printf("Enter the Year: ");
scanf("%d", &year);
Then, the user is asked to enter the value of the year.
// logic
if (year % 400 == 0){
printf("%d is a leap year", year);
}
else if (year % 100 == 0){
printf("%d is not a leap year.", year);
}
else if (year % 4 == 0){
printf("%d is a leap year.", year);
}
After taking the input, the logical part of the program starts. If the year is perfectly divisible by 4, then it will be a leap year. If the year is divisible by 4 and 100, then it won’t be a leap year.
In that case, it will only be a leap year if it is perfectly divisible by both 4 and 400.
Some of the used terms are as follow:
#include <stdio.h> – In the first line we have used #include, it is a preprocessor command that tells the compiler to include the contents of the stdio.h(standard input and output) file in the program.
The stdio.h is a file which contains input and output functions like scanf() and printf() to take input and display output respectively.
Int main() – Here main() is the function name and int is the return type of this function. The Execution of any Programming written in C language begins with main() function.
scanf() – scanf() function is used to take input from the user.
printf() – printf() function is used to display and print the string under the quotation to the screen.
% – It is known as Modulus Operator, used for division. It returns the remainder.
If. . . else – An if statement can be followed by an optional else statement, which executes when the Boolean expression is false. If Statement executes when the Boolean expression is True.
// – Used for Commenting in C.
Conclusion
I hope after reading this post, you understand to check whether a year is a leap year or not.
So, what are you waiting for, enter your birth year as input and check whether it’s a leap year or a normal year.
If you have any doubt regarding the topic, feel free to contact us in the Comment Section. We will be delighted to help you.