In this post, we will learn How to Multiply Two 8 Bit Numbers in 8085 Programming. Let’s understand about 8085 Microprocessors and Instruction Sets of 8085 Microprocessors then, it will be easy to code the 8085 Program to Multiply Two 8 Bit Numbers.
8085 Microprocessor
8085 microprocessor is an 8-bit microprocessor as it has an 8 bit ALU. 8085 microprocessor has a 16-bit address bus and an 8-bit data bus. The memory range of 8085 is 0000H to FFFFH. Each memory location can store 1 byte of data.
Now, let’s see the 8085 Program to Multiply Two 8 Bit Numbers.
8085 Program to Multiply Two 8 Bit Numbers
Problem
Write an 8085 Program to Multiply Two 8 Bit Numbers stored at location 2000H and 2001H and store the result at 2002H and 2003H.
LXI H, 0000H ;Result will be in HL, initialized to 0 LXI D, 0000H ;DE initialized to 0 LDA 2000H ;Take 1st operand ADI 00h ;For Zero Check JZ Store ;For Zero check MOV E,A ;DE = 1st Operand LDA 2001H ;Take 2nd Operand ADI 00H ;For zero check JZ Store ;For zero check MOV C,A ;C = 2nd operand Back : DAD D ;Add the 1st operand to HL,HL = HL + DE DCR C ;Decrement the 2nd operand JNZ Back ;Loop till C becomes 0 Store : SHLD 2002H ;Store the result HLT; ;End your Program
Output
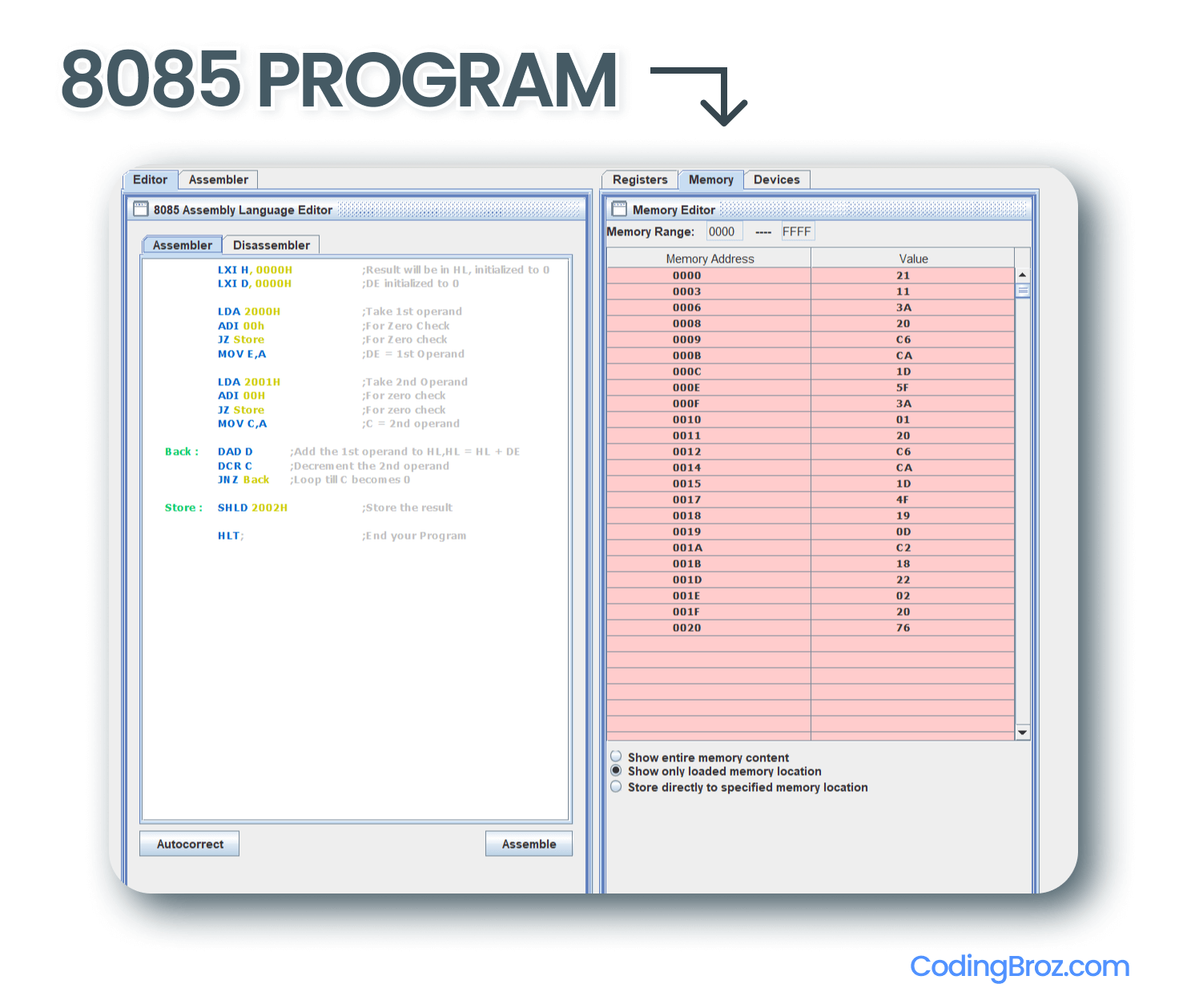
How Does This Program Work ?
Let’s see about all the instruction sets used to perform this 8085 program to multiply two 8 bit numbers.
Instruction Sets
- LXI – LXI is an immediate addressing mode. In this mode data is specified in the instruction itself.
For example,
LXI H, 0000H means, Move immediatily the value of 0000H into the register pair HL. i.e. HL <- 0000H - LDA 16-bit address – The accumulator is loaded with contents of the memory location having the address.
For example,
LDA 2000H, i.e. A <- [2000] - ADI 00H – It means Add immediately 00 with the content of accumulator and store the result in accumulator.
- JZ – It is condition jump instruction set in due to which the program jumps to the specified location given directly or given by a label, if the condition is True.
JZ 2000H, i.e. PC <- 2000H, if Z = 1 - MOV B, C – It means move the contents of C register to B register. i.e. B <- C
- DAD Rp(Register Pair) – This instruction adds the content of the given register pair with HL pair and stores the results in HL pair.
For example,
DAD B, i.e. HL <- HL + BC - DCR C, is a decrementer intruction which decrements the value of the given register by 1. e.g. DCR C, i.e. C <- C – 1
- SHLD 16-bit address – The HL pair is stored into the location pointed by the given address and address + 1.
for example,
SHLD 2002H, i.e. [2002]&[2003] <- H , i.e. [2002]<-L & [2003]<-H
Now, let’s understand the logic of the 8085 Program to Multiply Two 8-bit Numbers.
LXI H, 0000H ;Result will be in HL, initialized to 0 LXI D, 0000H ;DE initialized to 0
In this program, we have initialized the HL register pair and the DE register pair with 0.
LDA 2000H ;Take 1st operand ADI 00h ;For Zero Check JZ Store ;For Zero check MOV E,A ;DE = 1st Operand
Then, we take using LDA to take the input from location 2000H, and then we use conditional jump.
LDA 2001H ;Take 2nd Operand ADI 00H ;For zero check JZ Store ;For zero check MOV C,A ;C = 2nd operand
Then, we take using LDA to take the input from location 2001H, and then we use conditional jump.
Back : DAD D ;Add the 1st operand to HL,HL = HL + DE DCR C ;Decrement the 2nd operand JNZ Back ;Loop till C becomes 0
Then, using DAD D instruction HL stores the value of HL + DE register pair value, and then we decrement the value of C register by 1 for loop till it becomes 0.
Store : SHLD 2002H ;Store the result
Then, we store the value in the 2002H location using the SHLD 2002H instruction.
This is the 8085 Program to Multiply Two 8-bit Numbers.
Conclusion
I hope after going through this post, you understand how to code the 8085 Program to Multiply Two 8-bit Numbers.
If you have any doubt regarding the topic, feel free to contact us in the Comment Section. We will be delighted to help you.
Also Read: