In this post, we will learn how to calculate kinetic energy of an object using C Programming language.
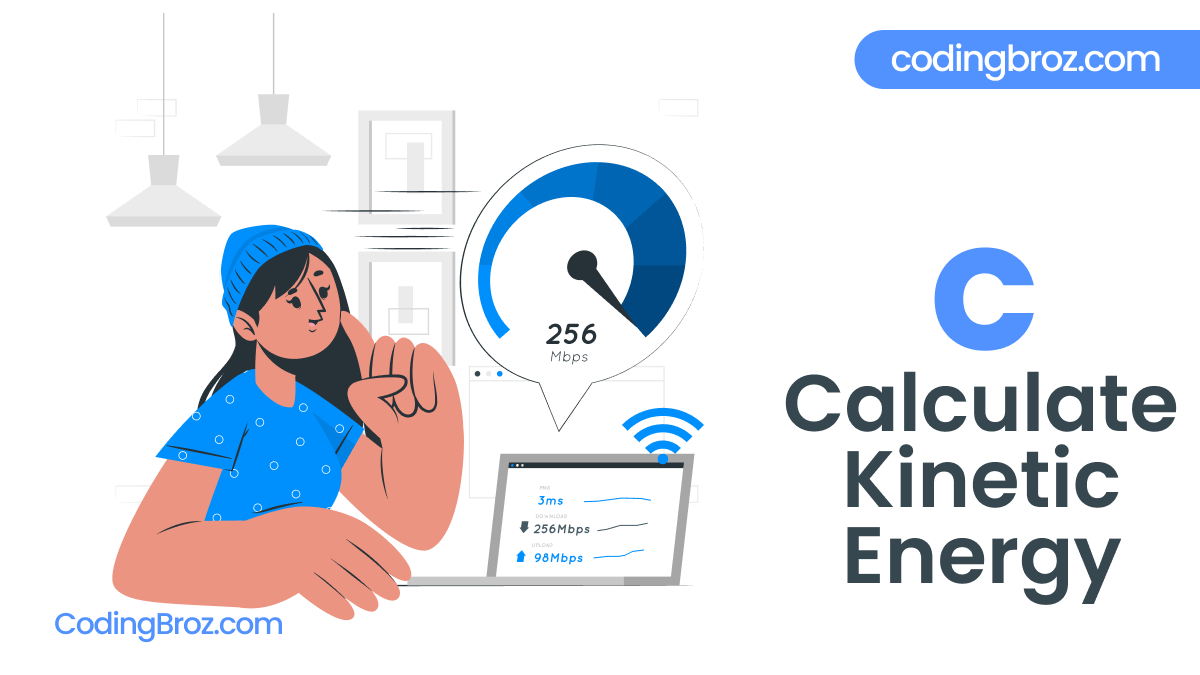
Kinetic Energy is the energy of an object when moving from the state of rest to motion. The Formula to calculate kinetic energy of an object is
Kinetic Energy = ½ x Mass x (Velocity)2
We will be using the same formula in our program to compute the kinetic energy of an object.
So, without further ado, let’s begin this tutorial.
C Program To Calculate Kinetic Energy
// C Program To Calculate Kinetic Energy #include <stdio.h> int main(){ float mass, velocity, KE; // Asking for Input printf("Enter the mass of the object(in Kg): "); scanf("%f", &mass); printf("Enter the velocity of the object(in m/s): "); scanf("%f", &velocity); // Calculating Kinetic Energy if (mass > 0){ KE = (0.5 * mass * velocity * velocity); printf("The Kinetic Energy of the object is %.2lf J.", KE); } else{ printf("Mass cannot be Negative."); } return 0; }
Output
Enter the mass of the object(in Kg): 25
Enter the velocity of the object(in m/s): 5
The Kinetic Energy of the object is 312.50 J.
How Does This Program Work ?
float mass, velocity, KE;
In this program, we have declared three float data type variables named mass, velocity and KE.
// Asking for Input
printf("Enter the mass of the object(in Kg): ");
scanf("%f", &mass);
printf("Enter the velocity of the object(in m/s): ");
scanf("%f", &velocity);
Then, the user is asked to enter the values of mass and velocity.
// Calculating Kinetic Energy
if (mass > 0){
KE = (0.5 * mass * velocity * velocity);
printf("The Kinetic Energy of the object is %.2lf J.", KE);
}
else{
printf("Mass cannot be Negative.");
}
Now, we calculate the kinetic energy of the object using the formula ½ x Mass x (Velocity)2.
Note: Here, we have used if . . . else statement because there is no kinetic energy of an object having negative mass.
C Program To Calculate Kinetic Energy Using pow() function
// C Program To Calculate Kinetic Energy Using pow() function #include <stdio.h> #include <math.h> int main(){ float mass, velocity, KE; // Asking for Input printf("Enter the mass of the object(in Kg): "); scanf("%f", &mass); printf("Enter the velocity of the object(in m/s): "); scanf("%f", &velocity); // Calculating Kinetic Energy if (mass > 0){ KE = ((0.5) * mass * pow(velocity, 2)); printf("The Kinetic Energy of the object is %.2f J.", KE); } else{ printf("Mass cannot be Negative."); } return 0; }
Output
Enter the mass of the object(in Kg): 10
Enter the velocity of the object(in m/s): 20
The Kinetic Energy of the object is 2000.00 J.
Conclusion
I hope after going through this post, you understand how to calculate kinetic energy of an object using C Programming language.
If you have any doubt regarding the program, feel free to contact us in the comment section. We will be delighted to help you.
Also Read: