In this post, you will learn to swap two numbers in C Programming language using different methods.
Writing a Swapping Program in C Programming language is a good try for logic building. You get to know different aspects of logic building and how to implement them in your program to get the desirable output.
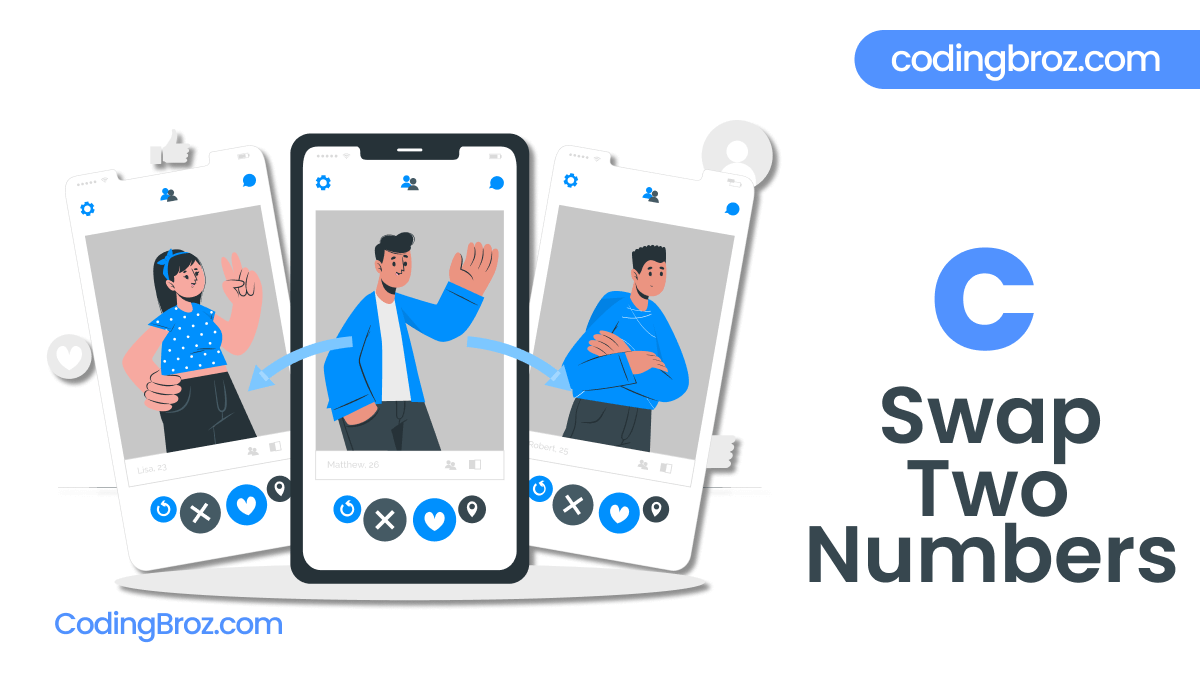
So, Without further ado, let’s begin the tutorial.
C Program To Swap Two Numbers
// C Program To Swap Two Numbers int main(){ double a, b, temp; // Storing first Number printf("Enter First Number: "); scanf("%lf", &a); // Storing Second Number printf("Enter Second Number: "); scanf("%lf", &b); // Values Swapping temp = a; // Value of a assigned to temp a = b; // Value of a assigned to b b = temp; // Value of b assigned to temp // Displays Output printf("First Number after Swapping is: %.2lf \n", a); printf("Second Number after Swapping is: %.2lf", b); return 0; }
Output
Enter First Number: 3.87
Enter Second Number: 5.85
First Number after Swapping is: 5.85
Second Number after Swapping is: 3.87
How Does This Program Work ?
double a, b, temp;
In this example, we have declared three variables named as a, b and temp.
// Storing first Number
printf("Enter First Number: ");
scanf("%lf", &a);
// Storing Second Number
printf("Enter Second Number: ");
scanf("%lf", &b);
Then, the user is asked to enter the first and second number which they want to swap.
// Values Swapping
temp = a; // Value of a assigned to temp
a = b; // Value of a assigned to b
b = temp; // Value of b assigned to temp
After that, temp variable is assigned the value of a variable. Then, the value of a variable is assigned to the b variable.
And finally the temp variable( which holds the value of a variable) is assigned to b.
This completes our swapping logic.
// Displays Output
printf("First Number after Swapping is: %.2lf \n", a);
printf("Second Number after Swapping is: %.2lf", b);
And after all these, the output is printed using printf() function. Here, we have used %.2lf because we want the program to show only till 2 decimal places.
Some used terms are as follow:
#include <stdio.h> – In the first line we have used #include, it is a preprocessor command that tells the compiler to include the contents of the stdio.h(standard input and output) file in the program.
The stdio.h is a file which contains input and output functions like scanf() and printf() to take input and display output respectively.
Int main() – Here main() is the function name and int is the return type of this function. The Execution of any Programming written in C language begins with main() function.
scanf() – scanf() function is used to take input from the user.
printf() – printf() function is used to display and print the string under the quotation to the screen.
// – Used for Commenting in C
Swap Numbers Without Using Temporary Variable
// C Program To Swap Two Numbers #include <stdio.h> int main(){ double a, b; // Storing first Number printf("Enter First Number: "); scanf("%lf", &a); // Storing Second Number printf("Enter Second Number: "); scanf("%lf", &b); // Swapping logic a = a - b; b = a + b; a = b - a; // Displays Output printf("First Number after Swapping becomes: %.2lf \n", a); printf("Second Number after Swapping becomes: %.2lf", b); return 0; }
Output
Enter First Number: 3.57
Enter Second Number: 7.43
First Number after Swapping becomes: 7.43
Second Number after Swapping becomes: 3.57
// Swapping logic
a = a - b;
b = a + b;
a = b - a;
Here, in this program instead of using a temporary variable, we have created a simple logic to swap two numbers.
Conclusion
I hope after going through this post, you get to understand how to swap two numbers in C Programming language.
If you still have any doubt regarding this, feel free to contact us in the Comment Section. We will be delighted to help you.